如何利用Python实现Floyd-Steinberg误差扩散抖动算法,以及该算法是如何提升图像质量的?
时间: 2024-11-01 17:16:10 浏览: 40
Floyd-Steinberg误差扩散抖动算法是一种常用的图像处理技术,它能够将连续色调的图像转换为具有较少颜色的半色调图像,从而优化图像质量。要实现这一算法,可以使用Python编程语言结合图像处理库Pillow或OpenCV来完成。以下是算法实现的步骤和代码示例:
参考资源链接:[Python实现图像抖动技术:误差扩散与Floyd-Steinberg算法](https://wenku.csdn.net/doc/2xtsg3d6do?spm=1055.2569.3001.10343)
首先,需要理解Floyd-Steinberg算法的核心思想:对图像进行遍历,每个像素的误差根据一个确定的矩阵分布到周围的像素上。这个算法通过减少量化误差,使得图像的视觉效果更佳细腻,避免了块状效应。
下面是利用Python实现Floyd-Steinberg算法的一个简化示例代码:
```python
from PIL import Image
import numpy as np
def floyd_steinberg_dithering(image):
# 将图像转换为灰度图像
image = image.convert('L')
width, height = image.size
img_array = np.array(image)
for i in range(height):
for j in range(width):
oldPixel = img_array[i][j]
newPixel = 255 if oldPixel > 127 else 0
img_array[i][j] = newPixel
quant_error = oldPixel - newPixel
if j + 1 < width:
img_array[i][j + 1] += quant_error * 7 / 16
if i + 1 < height:
if j - 1 >= 0:
img_array[i + 1][j - 1] += quant_error * 3 / 16
img_array[i + 1][j] += quant_error * 5 / 16
if j + 1 < width:
img_array[i + 1][j + 1] += quant_error * 1 / 16
# 重新创建图像并保存
dithered_image = Image.fromarray(img_array, 'L')
dithered_image.save('dithered_image.png')
# 载入图像并应用Floyd-Steinberg抖动算法
original_image = Image.open('original_image.jpg')
floyd_steinberg_dithering(original_image)
```
在这个实现中,我们首先将图像转换为灰度格式,然后遍历每个像素点,根据原始像素值与新像素值之间的差值来计算误差。随后,我们将计算出的量化误差按照Floyd-Steinberg算法定义的权重分配到周围像素上。这样,原本较大的量化误差就被分散到周围的像素中,从而减少了图像中的噪点和块状效应,提高了图像的整体质量。
为了获得更深入的理解,建议阅读《Python实现图像抖动技术:误差扩散与Floyd-Steinberg算法》。该资源详细讲解了算法的原理、实现方法,并提供了Python代码示例。通过学习该资源,可以更全面地掌握Floyd-Steinberg算法的实现,并理解其在图像质量优化方面的作用。
参考资源链接:[Python实现图像抖动技术:误差扩散与Floyd-Steinberg算法](https://wenku.csdn.net/doc/2xtsg3d6do?spm=1055.2569.3001.10343)
阅读全文
相关推荐
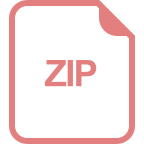
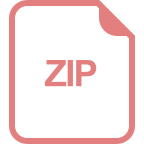


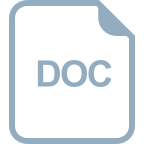
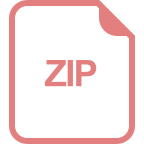
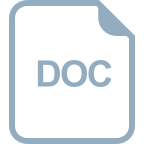

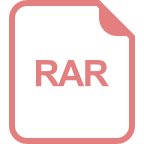
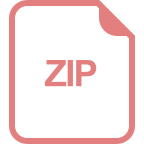
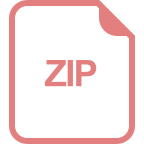
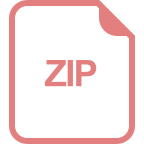
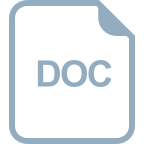
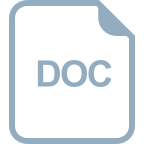


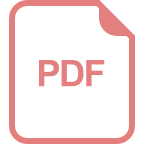