用Java实现数字时钟和正弦波动画模拟在Java编程中,创建图形用户界面(GUI)和动画效果。
时间: 2024-12-26 19:29:51 浏览: 0
在Java编程中,实现数字时钟和正弦波动画通常会涉及到Swing或JavaFX库,它们都是Java标准库的一部分,用于构建GUI(图形用户界面)。以下是简要步骤:
**数字时钟**:
1. 导入`javax.swing`包下的`JLabel`、`javax.swing.Timer`等组件。
2. 创建一个`JPanel`作为容器,并添加一个`JLabel`显示当前时间。
3. 使用`Timer`定时刷新`JLabel`的时间,通过`SimpleDateFormat`获取并更新显示。
4. 调整布局管理器以保证时钟组件的位置和样式。
```java
import javax.swing.*;
import java.awt.*;
import java.text.SimpleDateFormat;
import java.util.Date;
public class DigitalClock extends JFrame {
private JLabel timeLabel;
public DigitalClock() {
setLayout(new FlowLayout());
timeLabel = new JLabel(getCurrentTime(), SwingConstants.CENTER);
add(timeLabel);
Timer timer = new Timer(1000, e -> updateTimeLabel());
timer.start();
}
private String getCurrentTime() {
Date now = new Date();
SimpleDateFormat format = new SimpleDateFormat("hh:mm:ss");
return format.format(now);
}
private void updateTimeLabel() {
timeLabel.setText(getCurrentTime());
}
public static void main(String[] args) {
EventQueue.invokeLater(() -> new DigitalClock().setVisible(true));
}
}
```
**正弦波动画**:
1. 使用JavaFX或Swing的`Timeline`类,它可以播放动画序列。
2. 创建一个`Line`或`Path`元素来绘制正弦曲线。
3. 定义一个`Interpolator`来改变正弦函数的周期。
4. 在`Timeline`上设置关键帧,逐步改变曲线的位置或属性(如线宽、颜色等)。
```java
import javafx.animation.AnimationTimer;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.scene.shape.Line;
import javafx.scene.shape.Path;
import javafx.stage.Stage;
public class SineWaveAnimation extends Application {
private AnimationTimer animationTimer;
private Line sineWave;
@Override
public void start(Stage primaryStage) {
// 初始化场景
Scene scene = new Scene(new StackPane(), 800, 600);
sineWave = createSineWave();
// 设置动画
animationTimer = new AnimationTimer() {
@Override
public void handle(long now) {
double progress = now / (1000 * 60); // 每分钟变化一次
sineWave.setYProperty(sineWave.getYProperty() + Math.sin(progress * Math.PI * 2));
scene.requestRender();
}
};
animationTimer.start();
primaryStage.setScene(scene);
primaryStage.show();
}
private Line createSineWave() {
// 创建初始正弦线...
}
public static void main(String[] args) {
launch(args);
}
}
```
阅读全文
相关推荐
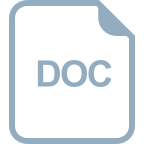
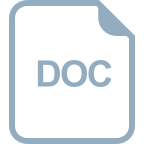
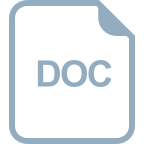
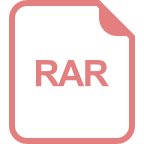
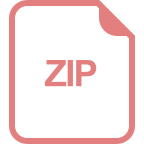
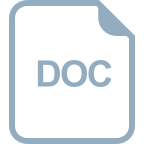
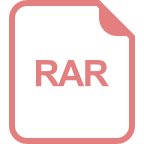
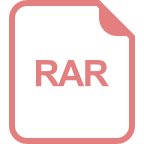
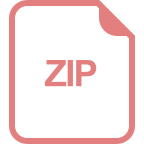
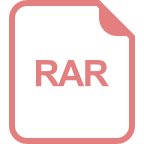
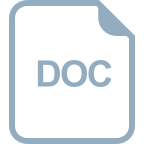
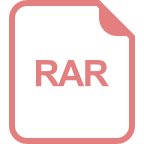
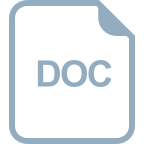
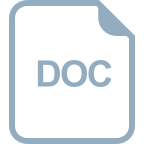
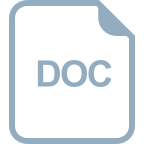
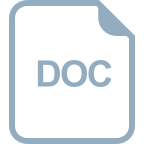
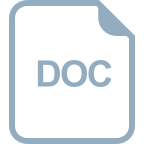
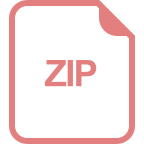