写一个代码,设置stdlib.h
时间: 2024-09-28 22:04:53 浏览: 23
在C语言中,`stdlib.h`包含了标准库的一些功能,例如内存管理、随机数生成等。然而,在C++环境中,`#include <cstdlib>`通常用于包含`cstdlib`库,它包含了`stdlib.h`中的一部分内容,尤其是与内存分配相关的函数。
如果你想要在C++程序中使用`std::malloc`和`std::free`这样的内存管理函数,你应该这么写:
```cpp
#include <cstdlib> // 引入cstdlib库
int main() {
// 使用 malloc 和 free 的例子
int* ptr = std::malloc(sizeof(int)); // 分配内存
if (ptr) {
*ptr = 42; // 对内存赋值
// ... 使用ptr...
std::free(ptr); // 释放内存
}
return 0;
}
```
这里要注意的是,在C++17之后,`std::malloc`和`std::free`已经被`std::memory_resource`和`std::allocator`替代,推荐使用现代的内存管理技术。如果你是在C++环境中,上述C风格的内存管理可能会引起编译警告。
相关问题
stdlib.h完整代码
抱歉,stdlib.h 是 C 标准库头文件之一,它包含了许多函数的声明和宏定义,但它本身并不是完整的代码。如果你需要使用 stdlib.h 的函数,你可以在你的代码中包含该头文件,并且链接相应的库文件。以下是一个使用 stdlib.h 的示例代码:
```
#include <stdlib.h>
#include <stdio.h>
int main() {
int *ptr = malloc(sizeof(int));
if (ptr == NULL) {
printf("Failed to allocate memory.\n");
return 1;
}
*ptr = 42;
printf("The value of ptr is %d\n", *ptr);
free(ptr);
return 0;
}
```
该程序使用了 stdlib.h 中的 malloc() 和 free() 函数来动态分配和释放内存。当内存分配失败时,程序会输出错误信息并退出。
#include<stdio.h> #include<stdlib.h> #include<string.h>
这是一段 C 语言的代码,包括了三个头文件:stdio.h、stdlib.h 和 string.h。其中 stdio.h 是标准输入输出头文件,提供了 printf 和 scanf 等函数;stdlib.h 是标准库头文件,提供了一些常用函数,如 malloc 和 free 等;string.h 是字符串头文件,提供了一些字符串处理函数,如 strlen 和 strcpy 等。这段代码的作用需要看后续的代码内容才能确定。
阅读全文
相关推荐
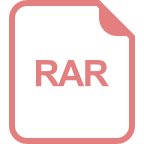
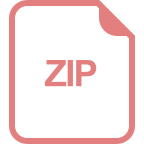
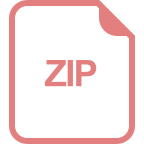


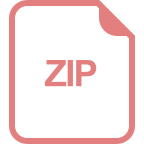
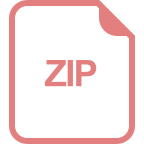
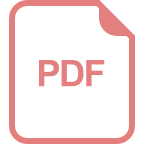
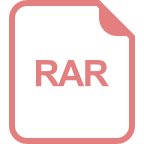
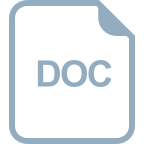
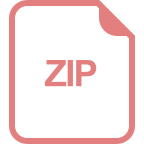
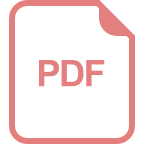
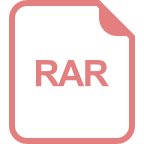
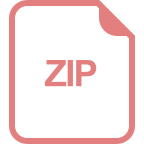
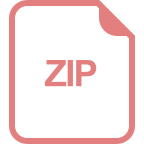
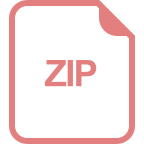