OpenGL实现正方体、球体实体几何造型完整代码
时间: 2025-01-02 10:48:38 浏览: 16
OpenGL是一种强大的图形库,用于渲染3D图形在计算机屏幕上。以下是使用OpenGL创建基本正方体和球体几何模型的基本步骤,这里我会提供一个非常基础的例子。请注意,实际应用中可能需要引入一些额外的库文件,并设置窗口和着色器等。
首先,我们需要包含必要的头文件:
```cpp
#include <GL/glut.h> // GLUT for window handling
#include <GL/gl.h> // OpenGL core functions
```
然后,我们定义一个顶点数据结构:
```cpp
struct Vertex {
float x, y, z;
};
```
接下来,定义正方体和球体的数据:
```cpp
const int VERTICES_CUBE[] = {...}; // 6 vertices for a cube (3 for each face)
const float CUBE_NORMALS[] = {...}; // normals for the cube faces
const int VERTICES_SPHERE[] = {...}; // vertex array for sphere
const float SPHERE_NORMALS[] = {...}; // normals for sphere
```
对于正方体:
```cpp
void drawCube() {
glBegin(GL_TRIANGLES); // start of triangles
for (int i = 0; i < 6; ++i) {
const auto &face = VERTICES_CUBE[i*4];
glVertex3fv(&face.x);
glVertex3fv(&face.y);
glVertex3fv(&face.z);
const auto &nextFace = VERTICES_CUBE[(i+1)%6*4];
glVertex3fv(&nextFace.x);
glVertex3fv(&nextFace.y);
glVertex3fv(&face.z);
}
glEnd(); // end of triangles
}
```
对于球体,由于OpenGL没有内置的球体绘制函数,通常会使用纹理映射或第三方库如GLM来辅助计算。这里只是一个简单的演示:
```cpp
void drawSphere(float radius) {
glPushMatrix();
glTranslatef(0, 0, -radius); // center the sphere
const int slices = 32, stacks = 32;
float angle = 0, increment = 2 * M_PI / slices;
float depth = 0, incrementZ = 1 / stacks;
glBegin(GL_TRIANGLE_STRIP);
for (int i = 0; i <= slices; ++i) {
float sinA = sin(angle);
float cosA = cos(angle);
glVertex3f(sinA * cosDepth, cosA * cosDepth, sinDepth);
angle += increment;
glVertex3f(sinA * cosDepth, cosA * cosDepth, -sinDepth);
depth -= incrementZ;
}
glEnd();
glPopMatrix();
}
```
最后,在主循环里调用这些函数:
```cpp
void display() {
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
drawCube();
drawSphere(1.f); // assuming a unit sphere
glutSwapBuffers();
}
// ...其他初始化和事件处理代码...
```
阅读全文
相关推荐






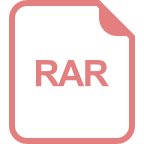









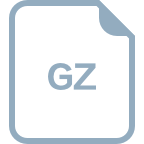

