python 操作HighScore Plus
时间: 2024-09-07 10:06:57 浏览: 53
HighScore Plus是一个假设的游戏高分插件或工具,它通常用于保存并管理游戏中的最高分数或其他成就数据。在Python中操作HighScore Plus可能会涉及到以下几个步骤:
1. **导入库**:如果你使用的是文本文件存储数据,你可以导入`json`库来进行读写操作。如果需要持久化存储,可能会用到`sqlite3`库。
```python
import json
# 或者
import sqlite3
```
2. **创建或打开数据库**(如果使用SQLite):
```python
conn = sqlite3.connect('highscores.db')
c = conn.cursor()
```
3. **添加新记录**:
```python
def add_high_score(player_name, score):
# 对于json:
with open('highscores.json', 'w') as f:
high_scores = json.load(f) if f.exists() else []
high_scores.append({'player': player_name, 'score': score})
json.dump(high_scores, f)
# 对于SQLite:
c.execute("INSERT INTO highscores (player, score) VALUES (?, ?)", (player_name, score))
conn.commit()
```
4. **查询最高分**:
```python
def get_top_score():
# json:
with open('highscores.json') as f:
high_scores = json.load(f)
return max(high_scores, key=lambda x: x['score'])['score']
# SQLite:
c.execute("SELECT MAX(score) FROM highscores")
top_score = c.fetchone()[0]
return top_score
```
5. **更新或删除记录**:
```python
def update_score(player_name, new_score):
# 更新json:
high_scores = json.load(open('highscores.json'))
for i, entry in enumerate(high_scores):
if entry['player'] == player_name:
high_scores[i]['score'] = new_score
break
json.dump(high_scores, open('highscores.json', 'w'))
# 更新SQLite:
c.execute("UPDATE highscores SET score=? WHERE player=?", (new_score, player_name))
conn.commit()
def delete_score(player_name):
# 删除json:
high_scores = json.load(open('highscores.json'))
high_scores = [entry for entry in high_scores if entry['player'] != player_name]
json.dump(high_scores, open('highscores.json', 'w'))
# 删除SQLite:
c.execute("DELETE FROM highscores WHERE player=?", (player_name,))
conn.commit()
```
阅读全文
相关推荐
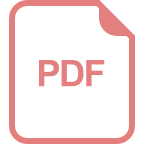
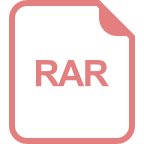

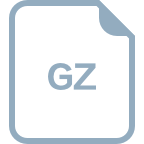
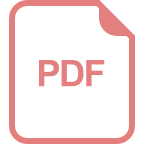
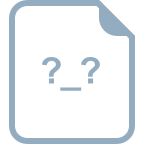
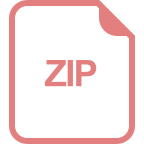
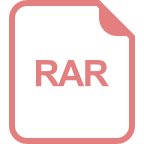
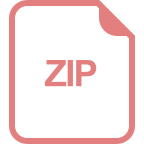
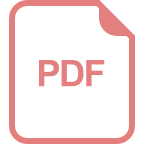
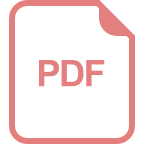
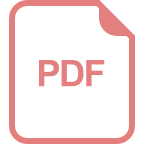
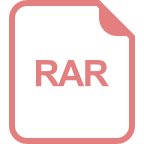