c语言万年历的源代码 在C语言中编写一个完整的万年历源代码会
时间: 2024-11-22 20:47:07 浏览: 4
在C语言中创建一个万年历的完整源代码涉及日期处理、时间管理和用户交互,下面是一个基础框架:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <stdbool.h> // 用于布尔值
// 定义日期结构
typedef struct {
int day;
int month;
int year;
} Date;
// 判断是否是闰年的函数
bool is_leap_year(int year) {
if (year % 4 != 0)
return false;
else if (year % 100 == 0)
return year % 400 == 0;
else
return true;
}
// 打印月份天数
void print_days_in_month(Date date) {
printf("%d", is_leap_year(date.year) ? 31 : 28); // 2月天数
for (int i = 3; i <= 12; ++i) {
printf(" %d", days_in_month[i]);
}
printf("\n");
}
// 其他月份的天数数组(假设非闰年)
const int days_in_month[] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
void main_calculator() {
// 获取用户输入
int year, month, day;
printf("请输入年份:");
scanf("%d", &year);
printf("请输入月份:");
scanf("%d", &month);
printf("请输入日期:");
scanf("%d", &day);
Date calendar_date = {day, month - 1, year}; // C语言月份从0开始计数
// 检查输入的有效性
if (calendar_date.month < 1 || calendar_date.month > 12 || calendar_date.day < 1 || calendar_date.day > days_in_month[calendar_date.month - 1]) {
printf("无效日期。\n");
return;
}
// 打印指定日期的月份日历
print_days_in_month(calendar_date);
print_calendar(calendar_date);
}
// 更详细的日历打印函数...
```
这个示例仅展示了基本的框架,实际的代码需要包括更完善的数据结构、错误处理以及打印功能。请注意,这个代码并没有处理所有边界情况和异常,例如用户输入非法年份时的处理。
阅读全文
相关推荐
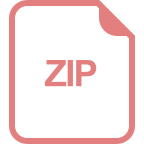
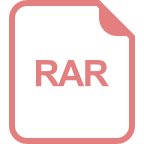
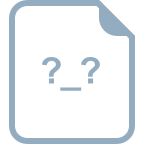
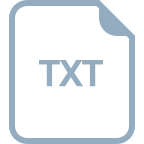
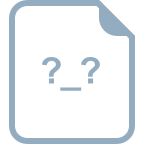
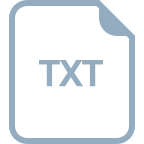
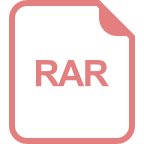
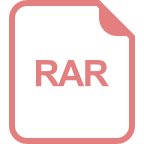
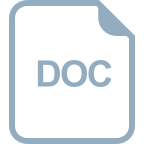
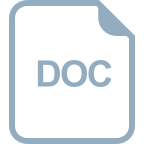
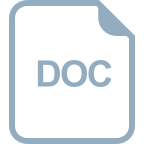
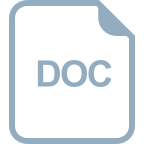


