HTML,页面显示“如果不做任何操作,5秒后自动返回首页”,其中的5秒呈现倒计时,当归零时自动跳转到另一个页面,或者当点击页面上的“确认”按钮时直接跳转到指定页面。给出这个页面的详细代码,并详细解释
时间: 2024-09-14 22:09:50 浏览: 40
要实现您描述的功能,我们需要编写一个简单的HTML页面,并通过JavaScript来控制5秒倒计时以及页面跳转的行为。下面是一个实现这一功能的示例代码:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>倒计时跳转页面</title>
<script type="text/javascript">
// 设置页面跳转的倒计时时间(5秒)
var countdown = 5;
// 更新倒计时显示的函数
function updateCountdown() {
// 获取页面上的倒计时显示元素
var countdownDisplay = document.getElementById('countdown');
// 更新显示的时间
countdownDisplay.textContent = countdown;
// 时间减1
countdown -= 1;
// 如果倒计时结束,执行页面跳转
if (countdown <= 0) {
// 页面跳转到指定的URL
window.location.href = 'home.html'; // 假设首页为home.html
}
}
// 页面加载完成后启动倒计时
window.onload = function() {
// 每隔1秒执行一次updateCountdown函数
setInterval(updateCountdown, 1000);
// 获取页面上的确认按钮元素,并添加点击事件监听
var confirmButton = document.getElementById('confirmButton');
confirmButton.onclick = function() {
// 点击按钮时直接跳转到指定页面
window.location.href = 'home.html'; // 同样假设首页为home.html
};
};
</script>
</head>
<body>
<!-- 显示倒计时的元素 -->
<div id="countdown">5</div>
<!-- 确认按钮 -->
<button id="confirmButton">确认</button>
</body>
</html>
```
解释如下:
1. `meta charset="UTF-8"` 设置了页面的字符编码为UTF-8,确保页面能正确显示各种字符。
2. 页面中定义了一个名为 `updateCountdown` 的JavaScript函数,该函数用于更新页面上的倒计时显示,并在倒计时结束时将页面跳转到指定的URL(这里假设首页为`home.html`)。
3. `window.onload` 事件确保在页面加载完毕后执行倒计时。
4. `setInterval(updateCountdown, 1000)` 每隔1秒调用一次 `updateCountdown` 函数,实现倒计时效果。
5. 页面上有一个显示倒计时的 `<div>` 元素和一个确认按钮,按钮的 `onclick` 事件绑定了一个函数,当用户点击按钮时,页面会立即跳转到指定页面。
6. 这里的代码假设首页为 `home.html`,在实际使用时需要替换为实际的URL。
阅读全文
相关推荐
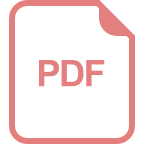
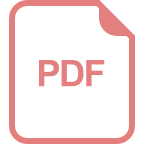
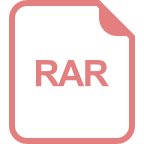



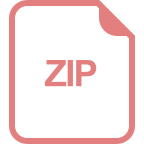
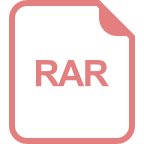
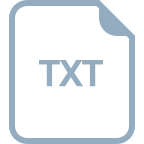
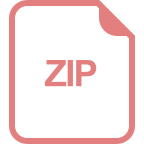
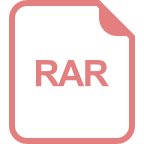
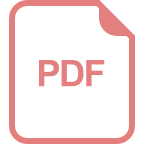