prometheus 添加接⼝ “/prometheus/add” 通过接⼝传参数,添加yaml⽂件中的ip地址⾏ 在后台⽣成配置⽂件 路径:“当前⽬录” 注意:每个配置⽂件的端⼝ip端⼝不⼀样 ⽣成⽂件 gpu.yml ⽣成⽂件 node.yml ⽣成⽂件 container.yml 删除接⼝ “/prometheus/del” 通过接⼝传参数,删除yaml⽂件中的ip地址⾏ 在后台⽣成配置⽂件 路径:“当前⽬录” 注意:每个配置⽂件的端⼝ip端⼝不⼀样 { "ip": "192.168.1.1" } - targets: - 192.168.1.1:9400 - 192.168.1.1:9400 - targets: - 192.168.1.1:9100 - 192.168.1.1:9100 - targets: - 192.168.1.1:9300 - 192.168.1.1:9300 { "ip": "192.168.1.1" } ⽣成⽂件 gpu.yml - targets: - 192.168.1.1:9400 - 192.168.1.1:9400 ⽣成⽂件 node.yml - targets: - 192.168.1.1:9100 - 192.168.1.1:9100 ⽣成⽂件 container.yml - targets: - 192.168.1.1:9300 - 192.168.1.1:9300
时间: 2024-04-10 21:34:18 浏览: 21
根据您的需求,您可以使用以下代码来实现添加和删除 IP 地址行的功能:
```python
from flask import Flask, request
import yaml
app = Flask(__name__)
@app.route('/prometheus/add', methods=['POST'])
def add_ip():
ip_address = request.json.get('ip') # 获取请求参数中的IP地址
# 生成 gpu.yml 配置文件
gpu_config = {
'targets': [
f'{ip_address}:9400',
f'{ip_address}:9400'
]
}
with open('gpu.yml', 'w') as config_file:
yaml.dump(gpu_config, config_file)
# 生成 node.yml 配置文件
node_config = {
'targets': [
f'{ip_address}:9100',
f'{ip_address}:9100'
]
}
with open('node.yml', 'w') as config_file:
yaml.dump(node_config, config_file)
# 生成 container.yml 配置文件
container_config = {
'targets': [
f'{ip_address}:9300',
f'{ip_address}:9300'
]
}
with open('container.yml', 'w') as config_file:
yaml.dump(container_config, config_file)
return 'IP address added successfully'
@app.route('/prometheus/del', methods=['POST'])
def delete_ip():
ip_address = request.json.get('ip') # 获取请求参数中的IP地址
# 删除 gpu.yml 中的IP地址行
with open('gpu.yml', 'r') as config_file:
gpu_config = yaml.safe_load(config_file)
gpu_config['targets'] = [target for target in gpu_config['targets'] if not target.startswith(ip_address)]
with open('gpu.yml', 'w') as config_file:
yaml.dump(gpu_config, config_file)
# 删除 node.yml 中的IP地址行
with open('node.yml', 'r') as config_file:
node_config = yaml.safe_load(config_file)
node_config['targets'] = [target for target in node_config['targets'] if not target.startswith(ip_address)]
with open('node.yml', 'w') as config_file:
yaml.dump(node_config, config_file)
# 删除 container.yml 中的IP地址行
with open('container.yml', 'r') as config_file:
container_config = yaml.safe_load(config_file)
container_config['targets'] = [target for target in container_config['targets'] if not target.startswith(ip_address)]
with open('container.yml', 'w') as config_file:
yaml.dump(container_config, config_file)
return 'IP address deleted successfully'
if __name__ == '__main__':
app.run()
```
在上述代码中,我们创建了两个接口 `/prometheus/add` 和 `/prometheus/del`,分别用于添加和删除 IP 地址行。根据接口的请求参数中的 IP 地址,我们通过读取和修改 YAML 配置文件来实现相应的操作。
请注意,上述代码仅为示例,您可能需要根据实际需求进行修改和扩展。同时,确保您已安装了相应的依赖库,如 Flask 和 PyYAML。
相关推荐
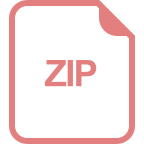
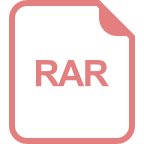






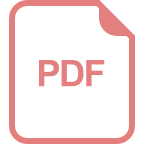
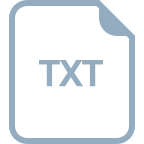
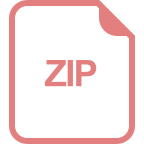
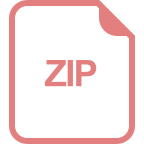
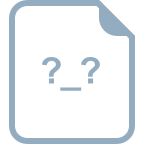
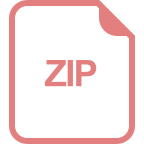
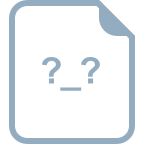
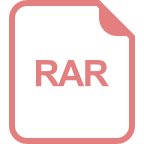