java利用字符串截取方法获取s8中小数点后面小数
时间: 2024-11-27 13:16:03 浏览: 0
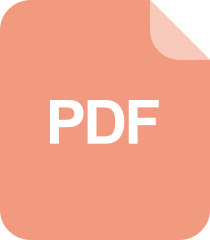
Java 判断字符为中文实例代码(超管用)
在Java中,如果你有一个字符串`s8`并且想获取其中小数点后的部分,你可以使用`substring()`方法结合`indexOf()`方法来完成这个任务。假设小数点位置已经被确定,例如通过查找`.`的位置,你可以这样做:
```java
String s8 = "这里是一个包含小数的字符串,如 123.456";
int decimalIndex = s8.indexOf('.'); // 找到小数点的位置
// 检查小数点是否存在
if (decimalIndex != -1) {
int start = decimalIndex + 1; // 小数点后面从下一个字符开始
String fraction = s8.substring(start); // 截取小数部分
System.out.println("小数部分: " + fraction);
} else {
System.out.println("字符串中不存在小数");
}
```
如果不确定小数点的位置,可以先尝试找到它,然后按照上述步骤操作。如果小数点不存在,`indexOf()`会返回-1,你需要处理这种情况。
阅读全文
相关推荐
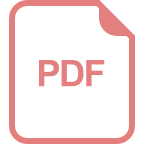
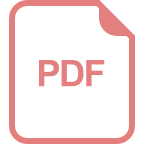
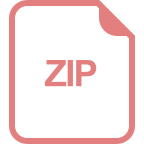
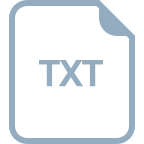
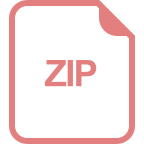
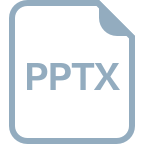
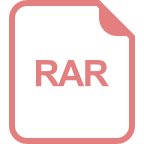
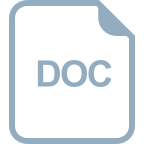
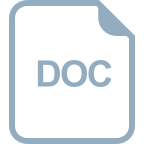
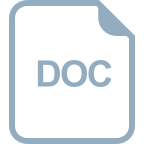







