Linux中用C++通过管道来获取命令行输出的内容
时间: 2024-04-14 08:26:09 浏览: 141
可以使用Linux中的管道(pipe)来获取命令行输出的内容。在C++中,可以通过fork()函数创建一个子进程,并在子进程中执行要获取输出的命令。然后,将子进程的标准输出重定向到管道的写端,父进程从管道的读端读取命令行输出。
下面是一个简单的示例代码:
```cpp
#include <iostream>
#include <unistd.h>
#include <sys/wait.h>
int main() {
int pipefd[2];
pipe(pipefd);
pid_t pid = fork();
if (pid == 0) {
// 子进程中执行命令并将输出写入管道
close(pipefd[0]); // 关闭管道的读端
dup2(pipefd[1], STDOUT_FILENO); // 将标准输出重定向到管道的写端
// 执行命令
execlp("your_command", "your_command", nullptr); // 替换为你要执行的命令
// 执行失败则退出
std::cerr << "Failed to execute command" << std::endl;
exit(1);
} else {
// 父进程从管道中读取命令行输出
close(pipefd[1]); // 关闭管道的写端
char buffer[4096];
ssize_t bytesRead;
while ((bytesRead = read(pipefd[0], buffer, sizeof(buffer))) > 0) {
// 处理读取到的数据,例如打印输出
std::cout.write(buffer, bytesRead);
}
// 等待子进程结束
int status;
waitpid(pid, &status, 0);
}
return 0;
}
```
在上面的代码中,需要将"your_command"替换为你要执行的命令。执行该程序时,它将创建一个子进程来执行命令,并将命令行输出通过管道传递给父进程,父进程则读取并处理输出。你可以根据需要对读取到的数据进行进一步处理或存储。
阅读全文
相关推荐
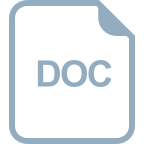
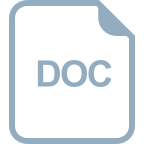
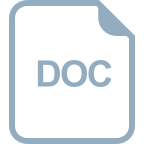
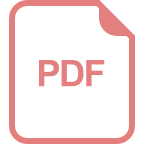
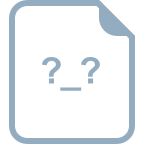
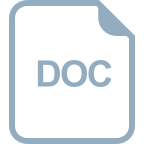
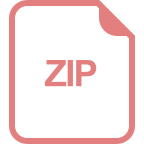
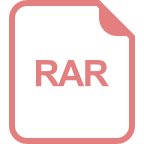
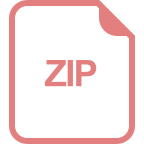
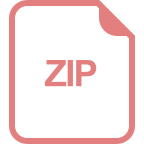
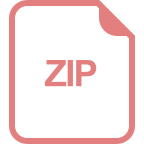
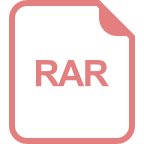
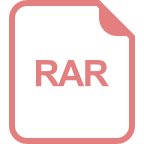
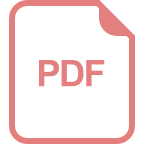
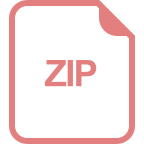
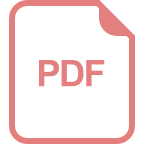