java excel设置下拉为树状数据
时间: 2024-04-23 22:27:25 浏览: 175
你可以使用Apache POI库来设置Excel下拉为树状数据。下面是一个示例代码:
```java
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.ss.util.CellRangeAddressList;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelTreeDropdownExample {
public static void main(String[] args) {
try (Workbook workbook = new XSSFWorkbook()) {
Sheet sheet = workbook.createSheet("Sheet1");
// 创建下拉列表的数据源
String[] items1 = {"Fruit", "Apple", "Banana", "Orange"};
String[] items2 = {"Color", "Red", "Blue", "Green"};
String[] items3 = {"Animal", "Dog", "Cat", "Bird"};
// 创建树状下拉列表的数据源
String[] treeItems = {"Category", "Fruit", "Color", "Animal"};
// 创建第一列的下拉列表
createDropdown(sheet, 0, 1, 10, items1);
// 创建第二列的下拉列表,绑定第一列的选中项
createDropdown(sheet, 1, 1, 10, items2);
sheet.addValidationData(createIndirectDataValidation(sheet, 1, 1, 10, 0));
// 创建第三列的下拉列表,绑定第二列的选中项
createDropdown(sheet, 2, 1, 10, items3);
sheet.addValidationData(createIndirectDataValidation(sheet, 2, 1, 10, 1));
// 设置第一行为树状下拉列表的数据源
sheet.addValidationData(createListDataValidation(sheet, 0, 0, 0, 3, treeItems));
// 保存Excel文件
workbook.write(new FileOutputStream("tree_dropdown.xlsx"));
} catch (IOException e) {
e.printStackTrace();
}
}
private static void createDropdown(Sheet sheet, int columnIndex, int firstRow, int lastRow, String[] items) {
DataValidationHelper dvHelper = sheet.getDataValidationHelper();
DataValidationConstraint dvConstraint = dvHelper.createExplicitListConstraint(items);
CellRangeAddressList addressList = new CellRangeAddressList(firstRow, lastRow, columnIndex, columnIndex);
DataValidation validation = dvHelper.createValidation(dvConstraint, addressList);
validation.setShowErrorBox(true);
sheet.addValidationData(validation);
}
private static DataValidation createIndirectDataValidation(Sheet sheet, int columnIndex, int firstRow, int lastRow, int refColumnIndex) {
DataValidationHelper dvHelper = sheet.getDataValidationHelper();
SheetNameFormatter sheetNameFormatter = dvHelper.createSheetNameFormatter("$B$2:$B$4");
String formula = sheetNameFormatter.format(sheet.getSheetName(), columnIndex + 1, columnIndex + 1);
DataValidationConstraint dvConstraint = dvHelper.createFormulaListConstraint(formula);
CellRangeAddressList addressList = new CellRangeAddressList(firstRow, lastRow, columnIndex, columnIndex);
DataValidation validation = dvHelper.createValidation(dvConstraint, addressList);
validation.setShowErrorBox(true);
return validation;
}
private static DataValidation createListDataValidation(Sheet sheet, int columnIndex, int firstRow, int lastRow, int refColumnIndex, String[] items) {
DataValidationHelper dvHelper = sheet.getDataValidationHelper();
DataValidationConstraint dvConstraint = dvHelper.createExplicitListConstraint(items);
CellRangeAddressList addressList = new CellRangeAddressList(firstRow, lastRow, columnIndex, columnIndex);
DataValidation validation = dvHelper.createValidation(dvConstraint, addressList);
validation.setShowErrorBox(true);
return validation;
}
}
```
这个示例代码会生成一个包含树状下拉列表的Excel文件。你可以根据自己的需求修改下拉列表的数据源和范围。请确保你已经添加了Apache POI库的依赖。
阅读全文
相关推荐
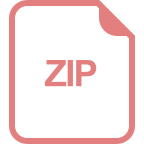
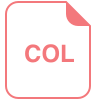
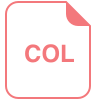
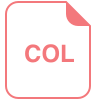
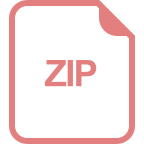
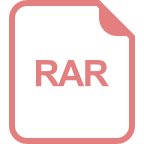