koa2 sequelize sqlite
时间: 2024-03-14 07:42:00 浏览: 35
Koa2是一个基于Node.js的Web开发框架,它使用了ES6的语法特性,提供了一种更简洁、更优雅的方式来构建Web应用程序。Sequelize是一个强大的Node.js ORM(Object-Relational Mapping)库,它提供了对多种数据库的支持,包括SQLite、MySQL、PostgreSQL等。SQLite是一种轻量级的嵌入式数据库引擎,它不需要独立的服务器进程,可以直接嵌入到应用程序中。
使用Koa2结合Sequelize和SQLite可以实现一个简单的Web应用程序。首先,你需要安装koa2、sequelize和sqlite3这三个npm包。然后,你可以创建一个Koa2应用程序,并配置Sequelize连接到SQLite数据库。接下来,你可以定义模型(Model)来映射数据库表,并使用Sequelize提供的API进行数据的增删改查操作。
下面是一个简单的示例代码:
```javascript
const Koa = require('koa');
const Sequelize = require('sequelize');
const app = new Koa();
// 配置Sequelize连接到SQLite数据库
const sequelize = new Sequelize({
dialect: 'sqlite',
storage: 'path/to/database.sqlite' // SQLite数据库文件路径
});
// 定义模型
const User = sequelize.define('user', {
name: Sequelize.STRING,
age: Sequelize.INTEGER
});
// 同步模型到数据库
sequelize.sync().then(() => {
console.log('Database synced');
}).catch((error) => {
console.error('Error syncing database:', error);
});
// 在路由中使用模型进行数据操作
app.use(async (ctx) => {
// 创建用户
const user = await User.create({ name: 'John', age: 25 });
// 查询用户
const users = await User.findAll();
// 更新用户
user.age = 26;
await user.save();
// 删除用户
await user.destroy();
ctx.body = users;
});
app.listen(3000, () => {
console.log('Server started on port 3000');
});
```
相关推荐
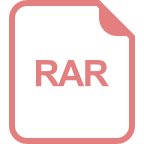
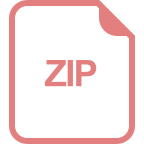














