采用C++语言来编写
时间: 2024-10-04 21:04:56 浏览: 31
在C++中,我们可以使用`cin`从标准输入流读取用户的输入,并使用`struct`或`class`来表示学生的信息。下面是一个简单的示例,展示了如何使用`std::vector`代替数组来动态管理学生数量:
```cpp
#include <iostream>
#include <vector>
#include <string>
// 定义学生类
class Student {
public:
std::string id;
int score;
// 构造函数
Student(std::string sid, int sc) : id(sid), score(sc) {}
};
int main() {
std::vector<Student> students; // 使用 vector 存储动态数量的学生
// 循环获取用户输入
int numStudents;
std::cout << "请输入学生数量(n<10): ";
std::cin >> numStudents;
if (numStudents >= 10) {
std::cerr << "错误:输入的学生数量大于等于10。\n";
return 1;
}
for (int i = 0; i < numStudents; i++) {
std::string idStr;
int score;
std::cout << "请输入第" << (i+1) << "个学生的学号(范围1000-9999): ";
std::cin >> idStr;
students.push_back(Student(idStr, score)); // 推荐使用构造函数接收输入
// 询问分数,这里简化为了直接赋值,实际应用应分开输入
std::cout << "请输入第" << (i+1) << "个学生的成绩: ";
std::cin >> score;
students.back().score = score; // 设置学生的分数
}
// 输出学生信息
std::cout << "\n学生信息:\n";
for (const auto& student : students) {
std::cout << "学号 " << student.id << ", 成绩 " << student.score << '\n';
}
return 0;
}
```
这段代码首先定义了一个学生类`Student`,接着通过`std::vector`来存储学生列表,循环获取用户输入,构建学生对象并添加到`students`中。最后遍历`students`输出所有学生信息。
阅读全文
相关推荐
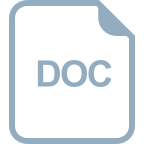
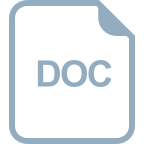
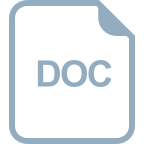
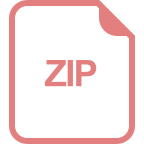
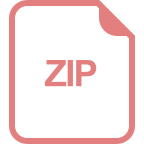
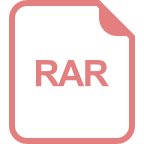
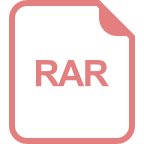
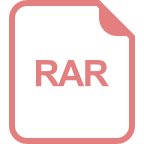
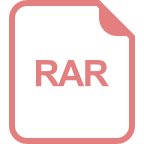
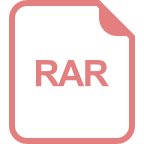
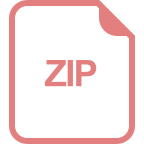
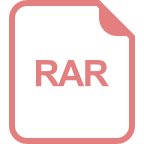
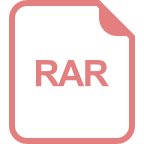
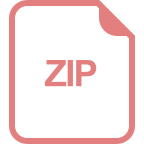
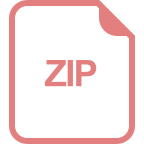
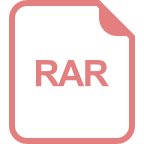
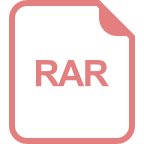