web自动化测试框架搭建封装
时间: 2023-12-10 07:33:41 浏览: 181
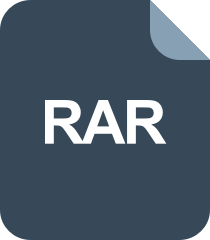
web自动化pom模式
根据提供的引用内容,我们可以了解到一个WEB UI自动化测试框架的三个核心模块:对象层、逻辑层和业务层。下面是一个简单的WEB UI自动化测试框架搭建封装的步骤:
1. 安装Python和Selenium库
```shell
pip install selenium
```
2. 创建一个基础类BasePage,用于封装一些基础方法,例如元素定位、点击、输入等操作。
```python
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.webdriver.common.by import By
class BasePage(object):
def __init__(self, driver):
self.driver = driver
def find_element(self, locator, timeout=10):
element = WebDriverWait(self.driver, timeout).until(EC.presence_of_element_located(locator))
return element
def click(self, locator):
element = self.find_element(locator)
element.click()
def input_text(self, locator, text):
element = self.find_element(locator)
element.clear()
element.send_keys(text)
```
3. 创建一个页面类,继承BasePage类,并实现该页面的元素定位和控件操作方法。
```python
from selenium.webdriver.common.by import By
from base_page import BasePage
class LoginPage(BasePage):
# 元素定位
username_input = (By.ID, 'username')
password_input = (By.ID, 'password')
login_button = (By.ID, 'login-button')
# 控件操作方法
def input_username(self, username):
self.input_text(self.username_input, username)
def input_password(self, password):
self.input_text(self.password_input, password)
def click_login_button(self):
self.click(self.login_button)
```
4. 创建一个逻辑层类,用于实现一些功能用例的模块。
```python
from page.login_page import LoginPage
class LoginAction(object):
def __init__(self, driver):
self.driver = driver
self.login_page = LoginPage(self.driver)
def login(self, username, password):
self.login_page.input_username(username)
self.login_page.input_password(password)
self.login_page.click_login_button()
```
5. 创建一个业务层类,用于实现测试用例的操作部分。
```python
from selenium import webdriver
from action.login_action import LoginAction
class TestLogin(object):
def setup_method(self):
self.driver = webdriver.Chrome()
self.driver.maximize_window()
self.driver.get('http://www.example.com')
def teardown_method(self):
self.driver.quit()
def test_login(self):
login_action = LoginAction(self.driver)
login_action.login('username', 'password')
# 添加断言等测试用例操作
```
阅读全文
相关推荐
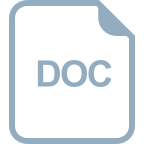
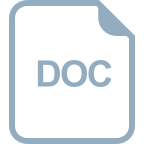
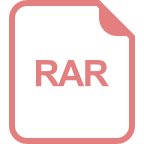
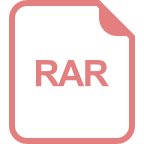
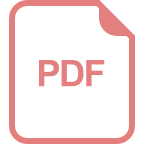
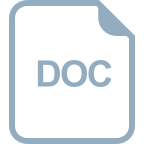
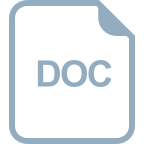
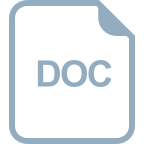
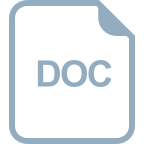
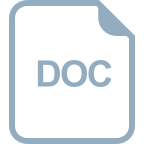
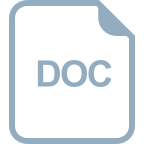
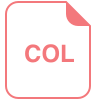
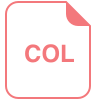
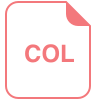
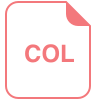
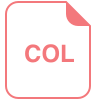
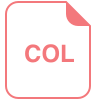
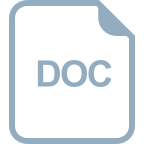