c语言中十进制转N进制的代码
时间: 2024-09-28 21:08:46 浏览: 21
在C语言中,将十进制数转换为任意基数(N进制)通常会涉及到整除和取余的过程,这可以借助循环来完成。下面是一个简单的函数示例,它使用了`itoa()`函数来实现字符串形式的十进制到N进制的转换,但这并不是原地转换:
```c
#include <stdio.h>
#include <stdlib.h>
// 注意这个函数并不适用于所有的N值,因为它依赖于库函数,仅限于0 <= N <= 36的情况
char* decimalToNthBase(int num, int base) {
if (base < 2 || base > 36)
return "Invalid base! Base should be between 2 and 36.";
char result[50]; // 预估最大位数为99999 (5 digits for a 5-digit number in base 36)
char* ptr = result;
while (num != 0) {
*ptr++ = '0' + num % base; // 将余数转换为字符并添加到结果前部
num /= base;
}
*ptr = '\0'; // 添加结束符
// 如果数字为负,需要在前面加上负号
if (num < 0) {
*--ptr = '-';
}
reverseString(ptr - 1, result); // 反转字符串,使得高位在前
return result;
}
void reverseString(char* start, char* end) {
while (start < end) {
char temp = *start;
*start = *end;
*end = temp;
start++;
end--;
}
}
int main() {
int decimal = 1234;
int base = 16; // 转换为十六进制
printf("Decimal %d in base %d is: %s\n", decimal, base, decimalToNthBase(decimal, base));
return 0;
}
```
在这个例子中,`decimalToNthBase`函数返回的是一个字符串表示法。如果需要精确的数值形式转换,可能需要自定义算法实现。
相关推荐
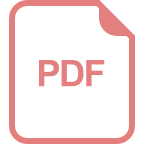
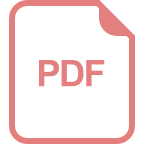
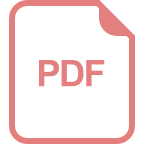

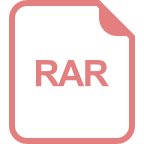
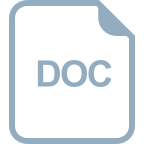
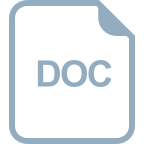
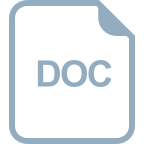
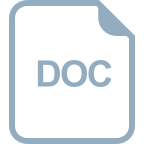








