C语言输入一串字符存入数组,输出字符串并计算这个字符串中字母的个数和大小写字母的个数
时间: 2024-10-14 11:10:40 浏览: 45
在C语言中,你可以通过以下步骤来输入一串字符并将它们存储到数组中,然后输出字符串,并统计其中字母的数量以及大写字母和小写字母的具体数目:
1. **输入字符串**:
```c
#include <stdio.h>
#define MAX_SIZE 100
int main() {
char str[MAX_SIZE];
printf("请输入一个字符串(不超过100个字符):");
fgets(str, MAX_SIZE, stdin); // 使用fgets读取字符串,避免换行符
}
```
2. **检查是否包含空格或其他非字母字符,并移除多余的换行符**:
```c
size_t len = strlen(str) - 1; // 去掉最后一个'\n'
str[len] = '\0'; // 将换行符替换为结束符
```
3. **统计字母总数、大写和小写字母的个数**:
```c
int alpha_count = 0;
int uppercase_count = 0;
int lowercase_count = 0;
for (size_t i = 0; i < len; i++) {
if (isalpha(str[i])) { // 检查是否为字母
alpha_count++;
if (isupper(str[i])) { // 如果是大写字母
uppercase_count++;
} else if (islower(str[i])) { // 如果是小写字母
lowercase_count++;
}
}
}
printf("原始字符串:%s\n", str);
printf("字母总数:%d\n", alpha_count);
printf("大写字母数:%d\n", uppercase_count);
printf("小写字母数:%d\n", lowercase_count);
}
```
4. **完整程序示例**:
```c
#include <stdio.h>
#include <ctype.h> // 包含处理字符类型的函数库
// ...
int main() {
// ...
size_t len = strlen(str) - 1;
str[len] = '\0';
int alpha_count = 0;
int uppercase_count = 0;
int lowercase_count = 0;
for (size_t i = 0; i < len; i++) {
if (isalpha(str[i])) {
alpha_count++;
if (isupper(str[i]))
uppercase_count++;
else if (islower(str[i]))
lowercase_count++;
}
}
printf("原始字符串:%s\n", str);
printf("字母总数:%d\n", alpha_count);
printf("大写字母数:%d\n", uppercase_count);
printf("小写字母数:%d\n", lowercase_count);
return 0;
}
```
阅读全文
相关推荐
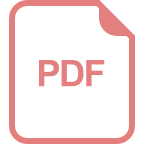
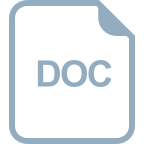
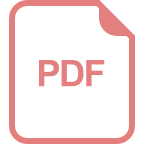
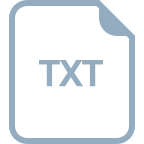
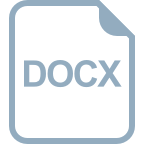
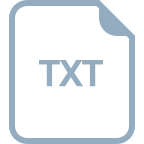
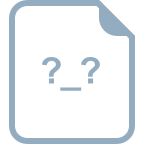
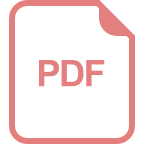
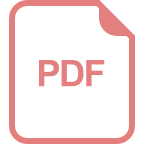
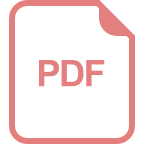
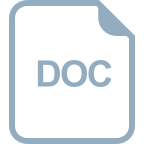


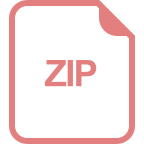
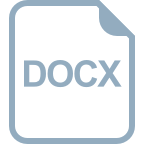
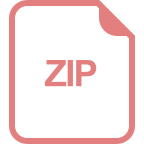
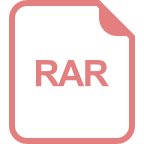