如何用C语言设计一个学生管理系统,该系统应包括哪些功能?例如,如何利用线性表数据结构来实现学生的插入、删除和修改操作,以及涉及到的文件操作步骤是什么?具体代码实现
时间: 2024-10-29 08:10:21 浏览: 33
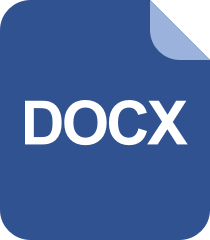
学生信息管理系统
设计一个简单的C语言学生管理系统,可以包含以下几个基本功能:
1. 学生信息管理:存储学生的姓名、学号、成绩等基本信息。
2. 插入操作:添加新的学生记录到系统中。
3. 删除操作:根据学号或其他标识符从系统中移除学生。
4. 修改操作:允许管理员更新现有学生的特定信息。
5. 查询操作:按名称、学号等搜索学生信息。
6. 文件操作:将数据保存至文件,以便持久化存储,并读取备份。
这里我们可以使用链表数据结构(如单向链表)来存储学生信息,因为插入、删除操作方便。首先定义一个`Student`结构体:
```c
typedef struct {
char name[50];
int id;
float score;
struct Student* next;
} Student;
```
接下来实现关键操作:
- 插入操作:
```c
void insert(Student** head, char* name, int id, float score) {
Student* newNode = (Student*)malloc(sizeof(Student));
newNode->name = malloc(strlen(name)+1);
strcpy(newNode->name, name);
newNode->id = id;
newNode->score = score;
newNode->next = *head;
*head = newNode;
}
```
- 删除操作:
```c
void delete(Student** head, int id) {
if (*head == NULL)
return;
if ((*head)->id == id) {
Student* temp = *head;
*head = (*head)->next;
free(temp);
return;
}
Student* current = *head;
while (current->next != NULL && current->next->id != id) {
current = current->next;
}
if (current->next != NULL) {
Student* temp = current->next;
current->next = current->next->next;
free(temp);
}
}
```
- 修改操作:
```c
void update(Student** head, int id, char* newName, float newScore) {
Student* current = *head;
while (current != NULL && current->id != id) {
current = current->next;
}
if (current != NULL) {
free(current->name);
current->name = malloc(strlen(newName) + 1);
strcpy(current->name, newName);
current->score = newScore;
}
}
```
为了实现文件操作,你需要一个打开、读取、写入和关闭文件的函数。这通常涉及到文件指针、文件路径和`fopen()`, `fprintf()`, 和 `fclose()` 等函数:
```c
void saveToFile(char* filename, Student* head) {
FILE* file = fopen(filename, "w");
// ... 写入学生信息到文件
fclose(file);
}
Student* loadFromFile(char* filename) {
FILE* file = fopen(filename, "r");
// ... 从文件读取并构造链表
fclose(file);
return head; // 返回新创建的链表头节点
}
```
阅读全文
相关推荐
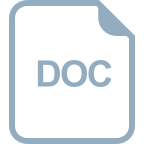
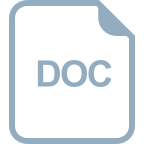
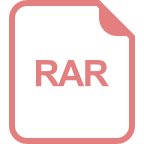
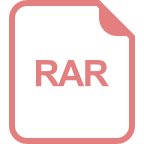
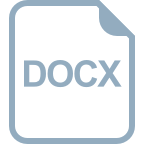
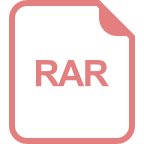
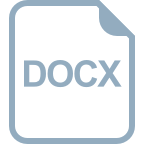
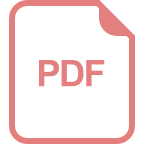
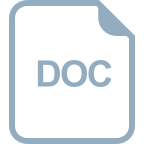
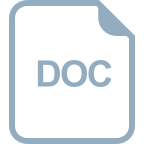
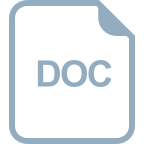
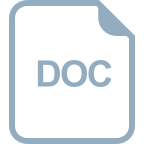
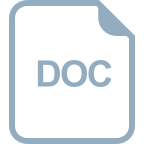
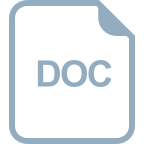



