c++游戏自动寻路代码
时间: 2024-09-06 18:02:03 浏览: 67
在C++中编写游戏自动寻路(Pathfinding)算法通常涉及到图论的概念,比如A*搜索、Dijkstra算法或BFS(广度优先搜索)。以下是一个简单的概述:
1. **创建地图数据结构**:使用二维数组或邻接矩阵表示游戏世界,其中每个节点代表可以移动的位置,边表示相邻节点间的可达路径。
2. **A*搜索算法**:这是一种启发式搜索,它结合了实际距离(cost)和估计到目标的距离(heuristic),计算出从起点到终点最短的路径。关键部分包括:计算F值(总成本),开放列表管理和关闭列表,以及“走一步看一步”的策略。
```cpp
struct Node {
int x, y;
int f, g, h; // cost from start, actual cost to current node, heuristic estimate
};
bool isValidMove(int x, int y) {
// 检查是否越界或不可通行
}
vector<Node> aStar(Node start, Node goal, vector<vector<int>>& grid) {
// 初始化节点,设置起始点
priority_queue<Node, vector<Node>, greater<Node>> openList;
openList.push(start);
start.g = start.h = 0;
while (!openList.empty()) {
Node current = openList.top(); openList.pop();
if (current.x == goal.x && current.y == goal.y) return reconstructPath(current);
// 遍历邻居并更新它们的状态
for (int dx, dy : {0, 0, -1, 1}) {
int nx = current.x + dx, ny = current.y + dy;
if (isValidMove(nx, ny)) {
Node neighbor = {nx, ny};
int tentative_g = current.g + 1;
// 如果找到更优路径,则更新状态
if (tentative_g < neighbor.g) {
neighbor.parent = ¤t;
neighbor.g = tentative_g;
neighbor.f = neighbor.g + neighbor.h;
openList.push(neighbor);
}
}
}
}
return {}; // 无法到达目标的情况
}
```
3. **路径重建**:当找到目标位置时,通过回溯`parent`指针重构路径。
阅读全文
相关推荐
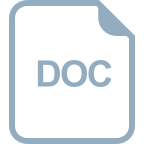
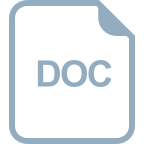
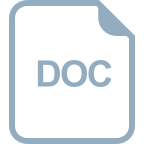

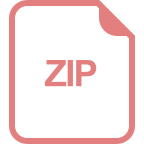
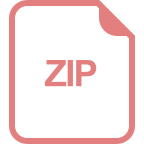
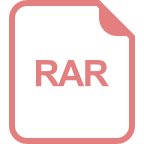


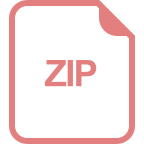
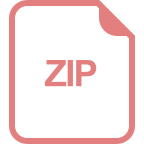
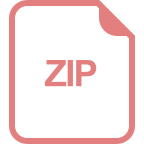
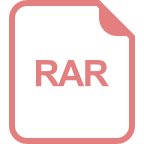
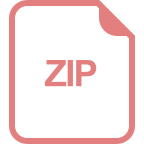
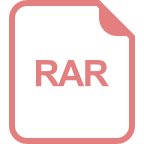
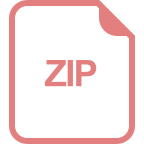
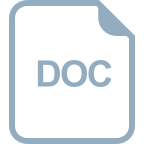
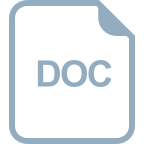