设计python程序 当ui界面按下某个按键,执行相关程序,若没有按键操作,则等待
时间: 2024-09-15 12:09:40 浏览: 42
在Python中,特别是在GUI编程中,比如Tkinter、PyQt等库,通常使用事件驱动模型来响应用户的输入,如按钮点击。下面是一个基本的例子,展示了如何在Tkinter中设计这样的程序:
```python
import tkinter as tk
def button_clicked():
# 这里是你想要执行的相关程序
print("Button clicked!")
# 或者,你可以在这里做更复杂的操作,比如调用函数、更新UI等
root = tk.Tk() # 创建主窗口
button = tk.Button(root, text="Click me", command=button_clicked) # 创建一个按钮
button.pack() # 将按钮添加到窗口上
# 如果没有按键操作,主循环会一直运行等待新的事件
root.mainloop()
```
在这个例子中,当用户点击"Click me"这个按钮时,`button_clicked`函数会被调用。如果没有按键操作,`mainloop()`方法将阻塞并持续监听用户交互,直到用户关闭窗口或者有新的事件发生。
如果你想让程序在一段时间内等待用户操作后自动执行一些操作,可以在主循环之外设置定时器,比如使用`threading`库:
```python
import time
import threading
def execute_after_delay():
# 按钮未点击时,延时后执行操作
time.sleep(5) # 可以根据实际需求调整时间
print("No button click detected, executing after delay.")
execute_thread = threading.Thread(target=execute_after_delay, daemon=True) # 设置为守护线程,防止主线程结束时导致异常
execute_thread.start()
root.mainloop()
```
在这个版本中,如果没有用户点击按钮,程序会在5秒后执行`execute_after_delay`函数。
阅读全文
相关推荐
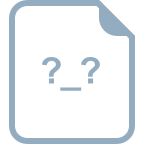
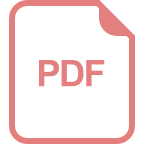
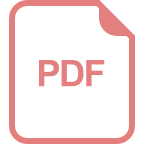















