《C语言程序设计》教材中,自行挑选4个代码示例,调试通过再提交。优先挑选关于数组、指针、结构体等内容的例子。
时间: 2024-10-10 07:05:05 浏览: 35
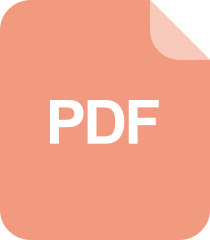
C语言 结构体数组详解及示例代码

当然,我可以帮你介绍几个涉及数组、指针和结构体的经典C语言代码示例。这些例子可以帮助你熟悉基本数据类型操作以及它们之间的关联。
**1. 数组示例:计算数组元素之和**
```cpp
#include <stdio.h>
int main() {
int arr[] = {1, 2, 3, 4, 5};
int sum = 0;
for (int i = 0; i < sizeof(arr) / sizeof(arr[0]); i++) {
sum += arr[i];
}
printf("Sum of array elements: %d\n", sum);
return 0;
}
```
在这个例子中,我们创建了一个整数数组并使用循环遍历数组,累加所有元素的值。
**2. 指针示例:动态内存分配和交换变量**
```cpp
#include <stdio.h>
void swap(int* a, int* b) {
int temp = *a;
*a = *b;
*b = temp;
}
int main() {
int x = 5, y = 10;
int* ptr_x = &x;
int* ptr_y = &y;
printf("Before swap: x = %d, y = %d\n", x, y);
swap(ptr_x, ptr_y); // 使用指针进行变量交换
printf("After swap: x = %d, y = %d\n", x, y);
return 0;
}
```
这个示例展示了如何使用指针进行变量值的交换,利用了指针的地址运算。
**3. 结构体示例:创建和操作自定义数据类型**
```cpp
#include <stdio.h>
// 定义结构体
struct Person {
char name[20];
int age;
};
int main() {
struct Person p1 = {"Alice", 25};
struct Person p2 = {"Bob", 30};
printf("p1: Name - %s, Age - %d\n", p1.name, p1.age);
printf("p2: Name - %s, Age - %d\n", p2.name, p2.age);
// 结构体指针操作
struct Person* ptr_p1 = &p1;
ptr_p1->age = 30; // 修改年龄
printf("After change: p1: Name - %s, Age - %d\n", p1.name, p1.age);
return 0;
}
```
这里定义了一个结构体`Person`,包含姓名和年龄,然后演示了如何创建结构体实例并对其进行操作。
**4. 动态数组(链表)示例:创建简单单向链表**
```cpp
#include <stdio.h>
#include <stdlib.h> // 对于malloc和free的头文件
// 链表节点定义
struct Node {
int data;
struct Node* next;
};
// 创建新节点
struct Node* createNode(int data) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = data;
newNode->next = NULL;
return newNode;
}
int main() {
struct Node* head = NULL;
struct Node* newNode = createNode(1);
newNode->next = createNode(2); // 追加节点
if (head == NULL) {
head = newNode;
} else {
struct Node* temp = head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
// 遍历链表
struct Node* curr = head;
while (curr != NULL) {
printf("%d ", curr->data);
curr = curr->next;
}
printf("\n");
return 0;
}
```
这段代码实现了简单的链表,包括节点创建、追加节点以及遍历链表。
阅读全文
相关推荐
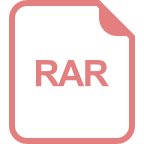
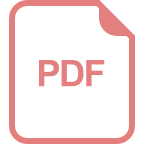
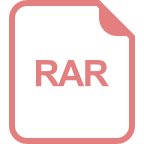
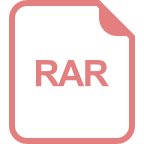
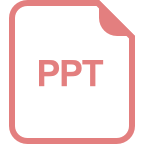
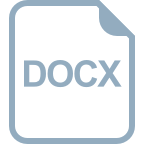
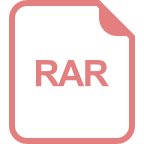
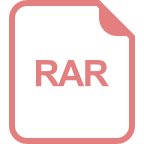
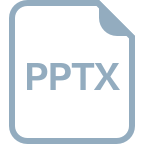
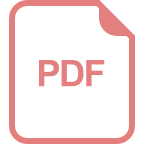
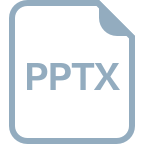
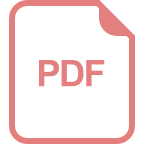
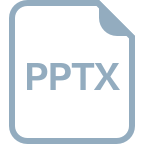
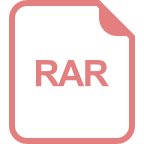