如何避免在Promise中发生 "index out of bounds" 错误?
时间: 2024-10-25 09:15:36 浏览: 34
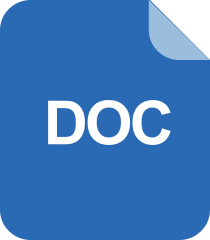
List index out of bounds(-1)错误解决办法
在JavaScript的Promise链中,"index out of bounds"错误通常发生在处理数组或其他索引依赖的数据结构时。为了避免这类错误,你可以采取以下几个步骤:
1. **检查数据有效性**:在访问数组元素之前,始终验证索引是否有效。可以使用`Array.prototype.length`属性检查索引是否小于数组长度。
```javascript
const index = i; // 这里假设i是你从外部获取的可能无效的索引
if (index >= 0 && index < array.length) {
const element = array[index];
// ...其他操作...
}
```
2. **使用?.运算符(可选链操作符)**:这个新的ES2020特性可以帮助你在访问嵌套属性或方法时防止`null`或`undefined`导致的错误。
```javascript
array?.[index]?.doSomething();
```
3. **Promise链中的错误处理**:在处理异步操作时,可以在`.then()`和`.catch()`块中捕获并处理可能出现的错误。
```javascript
fetchData()
.then((response) => response.json())
.then((data) => data[index])
.catch((error) => {
if (error instanceof ArrayIndexOutOfBoundsException) {
console.error('Index out of bounds error:', error);
} else {
console.error('Other error:', error);
}
});
```
4. **使用try/catch**: 对于不受`?.`支持的旧环境,可以使用传统的try/catch块来处理异常。
```javascript
try {
const element = array[index];
} catch (error) {
if (error instanceof TypeError && 'index' in error) {
console.error('Index out of bounds error:', error.message);
} else {
throw error;
}
}
```
阅读全文
相关推荐















