vue3全球 国家和地区的联动
时间: 2024-12-26 12:17:40 浏览: 8
### Vue3 实现全球国家和地区选择联动组件
为了实现Vue3中的全球国家和地区选择联动功能,可以创建一个自定义的下拉菜单组件来处理国家和地区的动态更新。下面是一个详细的实现方案:
#### 1. 安装依赖项
确保项目已经安装了必要的依赖包,如`axios`用于HTTP请求获取地区数据。
```bash
npm install axios vue-i18n
```
#### 2. 创建国家/地区数据源文件
准备一份JSON格式的数据文件 `countries.json` ,其中包含了各个国家及其对应的城市列表。
```json
[
{
"name": "China",
"cities": ["Beijing", "Shanghai"]
},
...
]
```
#### 3. 编写Vue 组件逻辑
编写名为 `CountryRegionSelector.vue` 的单文件组件,在此组件内部通过监听事件改变选项并触发相应的子区域加载。
```vue
<template>
<div class="selector">
<!-- Country Selector -->
<select v-model="selectedCountry" @change="onCountryChange()">
<option value="">Select a country</option>
<option v-for="(country, index) in countries" :key="index">{{ country.name }}</option>
</select>
<!-- Region/City Selector based on selected country -->
<select v-if="regions.length > 0" v-model="selectedCity">
<option value="">Choose city...</option>
<option v-for="(region, idx) in regions" :key="idx">{{ region }}</option>
</select>
</div>
</template>
<script setup lang="ts">
import { ref } from 'vue';
import axios from 'axios';
// Data properties initialization
const selectedCountry = ref('');
const selectedCity = ref('');
const countries = ref([]);
const regions = ref([]);
async function fetchCountries() {
try {
const response = await axios.get('/path/to/countries.json');
countries.value = response.data;
} catch (error) {
console.error('Error fetching countries:', error);
}
}
function onCountryChange() {
// Clear previous selection when changing the country.
selectedCity.value = '';
// Filter cities according to chosen country name.
const currentRegions = countries.value.find(
c => c.name === selectedCountry.value)?.cities || [];
regions.value = [...currentRegions];
}
fetchCountries();
</script>
```
该代码片段展示了如何构建一个简单的双向绑定的选择器结构,并利用JavaScript对象操作实现了基于所选国家自动填充城市的功能[^1]。
阅读全文
相关推荐
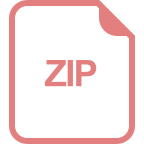
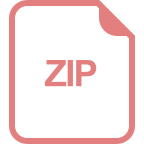
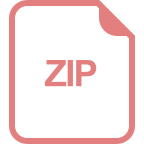
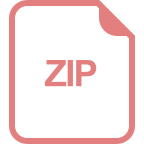
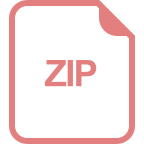
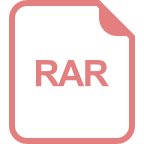
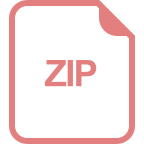
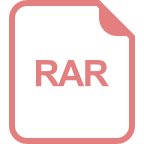
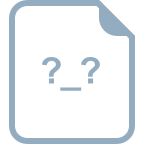
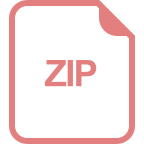
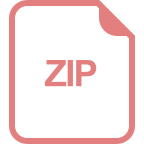
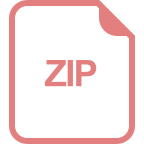
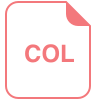
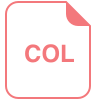


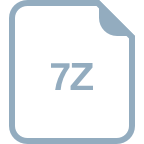
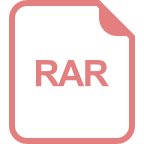