WPF 根据Grid宽高,拖曳图像区域
时间: 2024-02-04 17:30:19 浏览: 25
您可以使用WPF的拖拽功能来实现根据Grid的宽高来拖拽图像区域。下面是一个简单的示例代码:
```xml
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="*" />
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="*" />
</Grid.ColumnDefinitions>
<Image Source="your_image_source.jpg" Width="100" Height="100"
MouseDown="Image_MouseDown" MouseMove="Image_MouseMove" MouseUp="Image_MouseUp" />
</Grid>
```
在代码中,我们添加了一个Image元素,并为其添加了MouseDown、MouseMove和MouseUp事件处理程序。接下来,您可以在代码-behind文件中实现这些事件处理程序的逻辑。
```csharp
private bool isDragging = false;
private Point startPoint;
private void Image_MouseDown(object sender, MouseButtonEventArgs e)
{
isDragging = true;
startPoint = e.GetPosition(null);
((Image)sender).CaptureMouse();
}
private void Image_MouseMove(object sender, MouseEventArgs e)
{
if (isDragging && e.LeftButton == MouseButtonState.Pressed)
{
Point currentPoint = e.GetPosition(null);
double offsetX = currentPoint.X - startPoint.X;
double offsetY = currentPoint.Y - startPoint.Y;
Image image = (Image)sender;
double newLeft = Canvas.GetLeft(image) + offsetX;
double newTop = Canvas.GetTop(image) + offsetY;
// 限制图像区域在Grid内部
if (newLeft >= 0 && newLeft + image.ActualWidth <= grid.ActualWidth)
Canvas.SetLeft(image, newLeft);
if (newTop >= 0 && newTop + image.ActualHeight <= grid.ActualHeight)
Canvas.SetTop(image, newTop);
startPoint = currentPoint;
}
}
private void Image_MouseUp(object sender, MouseButtonEventArgs e)
{
isDragging = false;
((Image)sender).ReleaseMouseCapture();
}
```
在这段代码中,我们通过判断是否按下鼠标左键并且isDragging为true来判断是否处于拖拽状态。我们使用起始点和当前点的差异来计算图像的偏移量,并将其应用于图像的位置。同时,我们还添加了限制条件,确保图像区域不会超出Grid的范围。
请注意,这只是一个基本示例,您可能需要根据实际需求进行更多的逻辑处理。
相关推荐
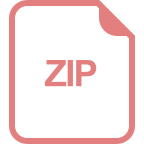
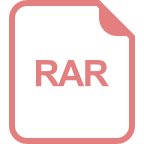














