用线性回归和多项式回归构建回归模型,并用网格搜索寻找最优超参数,给出Python代码
时间: 2024-11-10 09:13:52 浏览: 38
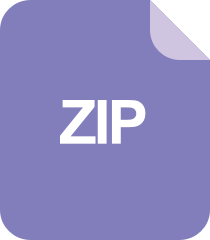
数据科学 机器学习系列5 利用Scikit-learn构建回归模型:准备和可视化数据.ipynb
在线性回归中,我们通常假设输入特征和输出之间存在线性关系。而多项式回归则是通过引入自变量的高次幂来拟合非线性的数据。下面是一个简单的例子,展示如何使用`sklearn`库进行线性和多项式回归,并使用网格搜索优化`PolynomialFeatures`和`LinearRegression`的参数:
```python
# 导入所需库
from sklearn.preprocessing import PolynomialFeatures
from sklearn.linear_model import LinearRegression
from sklearn.model_selection import GridSearchCV
import numpy as np
# 创建样本数据
np.random.seed(0)
X = np.linspace(-3, 3, 100)[:, np.newaxis]
y = X ** 2 + 2 * X + 1 + np.random.normal(0, 0.1, size=X.shape)
# 定义线性模型
model = LinearRegression()
# 定义多项式特征转换和参数网格
degree_values = [1, 2, 3] # 多项式阶数
poly_features = PolynomialFeatures(degree=degree_values, include_bias=False)
param_grid = {'poly_features__degree': degree_values}
# 组织网格搜索
grid_search = GridSearchCV(estimator=model, param_grid=param_grid, cv=5) # 交叉验证次数
# 训练模型
grid_search.fit(X, y)
# 获取最佳参数和对应的模型
best_degree = grid_search.best_params_['poly_features__degree']
best_model = grid_search.best_estimator_
# 打印最优参数和得分
print(f"Best degree: {best_degree}")
print(f"Best score: {grid_search.best_score_}")
# 使用最佳模型预测
X_test = np.linspace(-4, 4, 100)[:, np.newaxis]
y_pred = best_model.predict(X_test)
```
在这个代码中,我们首先创建了模拟的数据点,然后定义了一个线性回归模型。接着,我们创建了一个多项式特征转换器,并定义了一个度数网格。网格搜索会尝试所有这些度数,找到最优的模型。最后,我们展示了最优的多项式阶数以及在测试集上得到的最佳得分。
阅读全文
相关推荐
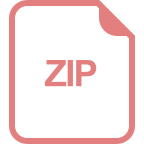
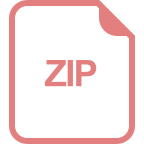















