C:\Users\drt\Desktop\上机2.cpp|56|error: no match for 'operator==' (operand types are 'std::__cxx11::string'
时间: 2024-10-04 13:04:59 浏览: 36
这个编译错误信息是在告诉你,在 C++ 文件 `C:\Users\drt\Desktop\上机2.cpp` 的第 56 行,有一个关于字符串(`std::__cxx11::string` 类型)的操作符 `==` 对比,找不到匹配的规则来进行比较。`std::__cxx11::string` 是 C++11 引入的 std 库中的标准字符串类型。
在 C++ 中,如果你想要比较两个字符串是否相等,你应该使用 `==` 操作符,就像这样:
```cpp
std::string str1 = "hello";
std::string str2 = "hello";
if (str1 == str2) {
// 如果两个字符串内容相同
}
```
但是,如果你试图将一个非字符串类型的变量与字符串比较,或者尝试对 `std::string` 和其他类型(比如整数)直接使用 `==`,就会出现编译错误,因为你没有提供正确的类型转换或重载的比较操作。
解决这个问题,你需要检查第 56 行的具体代码,看是否有意图比较字符串的地方,并确保所有参与比较的表达式都是 `std::string` 类型,或者进行适当的类型转换。可能是忘记包含头文件 `<string>`,或者错误地把其他类型的变量当作字符串处理了。
相关问题
C:\Program Files\CodeBlocks\yijvxingqiu\main.cpp|13|error: no match for 'operator==' (operand types are 'std::__cxx11::string' {aka 'std::__cxx11::basic_string<char>'} and 'char')|
根据错误提示,这个错误是因为你在比较一个 `std::__cxx11::string` 对象和一个 `char` 类型的值时使用了 `==` 运算符,但是这两个操作数的类型不匹配。
在 C++ 中,`std::__cxx11::string` 是表示字符串的数据类型,而 `char` 是单个字符的数据类型。这两种类型之间不能直接进行相等性比较。
如果你想比较一个 `std::__cxx11::string` 对象和一个字符,你可以将字符转换为字符串,然后再进行比较。例如:
```cpp
std::__cxx11::string str = "hello";
char ch = 'h';
if (str == std::__cxx11::string(1, ch)) {
// 执行操作
}
```
在上述代码中,我们使用 `std::__cxx11::string(1, ch)` 将字符 `ch` 转换为一个字符串对象,然后再与 `str` 进行比较。
请检查你的代码,确保你在比较字符串和字符时使用了正确的比较方式。如果仍然有问题,请提供更多相关代码,以便我可以更好地帮助你解决问题。
D:\aljbh2\Student_kechengsheji_1\Student_kechengsheji\Student_kechengsheji\Admin.cpp|255|error: no match for 'operator==' (operand types are 'int' and 'std::__cxx11::string {aka std::__cxx11::basic_string<char>}')|
这个错误是由于你在代码中比较了一个整数和一个字符串,导致编译器无法找到适合的 `operator==` 操作符进行比较。在 C++ 中,`operator==` 操作符只能用于相同类型的数据进行比较。为了解决这个问题,你需要确保你的比较操作中的数据类型相同。
如果你想要比较一个整数和一个字符串,你需要将整数转换为字符串,然后再进行比较。你可以使用 `std::to_string` 函数将整数转换为字符串,例如:
```cpp
int num = 1;
std::string str = "1";
if (std::to_string(num) == str) {
// do something
}
```
另外,如果你比较的是两个字符串,你需要确保它们的类型都是 `std::string`,例如:
```cpp
std::string str1 = "hello";
std::string str2 = "world";
if (str1 == str2) {
// do something
}
```
阅读全文
相关推荐
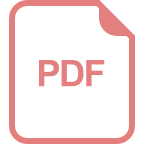
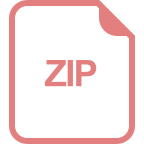
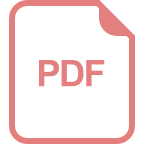













