用C语言,编程实现线性表两种存储结构中的基本操作的实现(线性表的创建 插入、删除和查找),并设计一个主菜单完成各个功能的调用。要求简单易懂一些
时间: 2024-11-06 08:35:35 浏览: 35
在C语言中,我们可以使用数组或链表这两种常见的数据结构来实现线性表。这里我们分别给出它们的基本操作实现以及一个简单的主菜单。
**一、数组实现**
1. **创建(初始化)**:
```c
#define SIZE 10 // 线性表大小
int linear_table[SIZE]; // 定义数组
void create_array(int* list, int size) {
for (int i = 0; i < size; ++i)
list[i] = 0; // 初始化所有元素为0
}
```
2. **插入**:
```c
void insert_array(int* list, int value, int index) {
if (index >= 0 && index < SIZE) {
for (int i = SIZE - 1; i > index; --i)
list[i] = list[i - 1];
list[index] = value;
} else {
printf("Error: Index out of range.\n");
}
}
```
3. **删除**:
```c
void delete_array(int* list, int index) {
if (index >= 0 && index < SIZE - 1) {
list[index] = list[index + 1];
} else {
printf("Error: Index out of range.\n");
}
}
```
4. **查找**:
```c
int search_array(const int* list, int value, int size) {
for (int i = 0; i < size; ++i)
if (list[i] == value)
return i;
return -1; // 如果没找到,返回-1
}
```
**二、链表实现**
对于链表,我们需要定义节点结构:
```c
typedef struct Node {
int data;
struct Node* next;
} Node;
```
然后实现类似的操作:
1. **创建(初始化)**:
```c
Node* create_linked_list() {
Node* head = NULL;
return head;
}
// 添加节点
void insert_linked_list(Node** head, int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = *head;
*head = newNode;
}
```
...
**三、主菜单**
```c
#include <stdio.h>
void main_menu() {
int choice;
while (1) {
printf("\n选择操作:\n1. 创建\n2. 插入\n3. 删除\n4. 查找\n5. 退出\n");
scanf("%d", &choice);
switch (choice) {
case 1: handle_create(); break;
case 2: handle_insert(); break;
case 3: handle_delete(); break;
case 4: handle_search(); break;
case 5: exit(0); // 退出程序
default: printf("Invalid choice.\n");
}
}
}
// 调用对应的函数处理用户的选择
void handle_create() { ... }
void handle_insert() { ... }
void handle_delete() { ... }
void handle_search() { ... }
// 其他函数...
```
以上就是C语言中线性表的创建、插入、删除和查找操作的示例,以及一个简单的主菜单设计。请注意,链表的插入和删除操作通常更高效,因为无需移动大量元素。
阅读全文
相关推荐
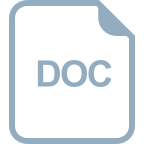
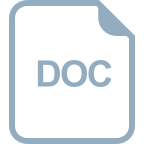
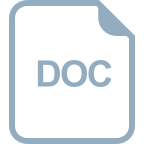






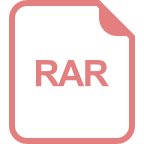
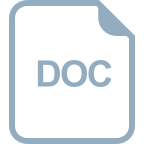
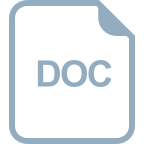
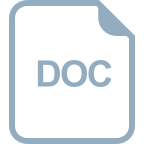
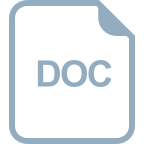
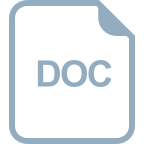
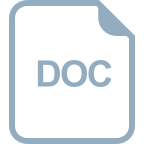


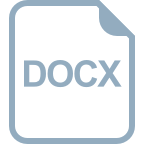