js 截取关键词from后第一个空格和第二个空格间的字符串?
时间: 2024-11-06 20:36:40 浏览: 9
在JavaScript中,你可以使用正则表达式结合`match()`函数来截取从"from"后的第一个和第二个空格之间的字符串。假设你的关键词是在一个字符串中的,例如:
```javascript
let str = "This is a sample string with 'from' being the keyword here.";
const keywordPattern = /from\s+(\S+)\s+/; // 正则匹配 "from" 后的第一个空格到第二个空格
// 使用match()获取匹配结果
let matchResult = str.match(keywordPattern);
if (matchResult) {
const keyword = matchResult[1]; // 第二个捕获组,即第一个空格后的部分
console.log("Keyword: ", keyword);
} else {
console.log("Keyword not found.");
}
```
这里,`\S+`表示匹配一个或多个非空白字符,所以`(\S+)`会捕获第一个空格后的连续非空白字符,直到遇到第二个空格。
相关问题
用户C语言判断字符串中有4个空格 ,如果有并截取第二个空格和第三个空格之间的字符串
在C语言中,要判断字符串中是否有四个空格,并截取从第二个空格到第三个空格之间的内容,你需要先遍历字符串,查找空格的数量,然后找到空格的位置。下面是一个示例程序,展示了如何完成这个任务:
```c
#include <stdio.h>
#include <string.h>
char* find_substring_with_spaces(char* str) {
int space_count = 0;
size_t len = strlen(str);
size_t start_index = -1, end_index = -1;
for (size_t i = 0; i < len; i++) {
if (str[i] == ' ') {
space_count++;
if (space_count == 4) { // 找到了第四个空格
start_index = i + 1; // 第二个空格的位置加一
break;
}
} else if (start_index != -1 && space_count > 4) { // 已经找到了三个空格并且又找到一个非空格
end_index = i;
break;
}
}
if (end_index == -1 || start_index == -1) {
return NULL; // 没有找到符合条件的子串
} else {
// 截取子串并返回指针
char* substr = malloc((end_index - start_index + 1) * sizeof(char));
strncpy(substr, str + start_index, end_index - start_index + 1);
substr[end_index - start_index] = '\0'; // 添加终止符
return substr;
}
}
int main() {
char str[] = "This is a string with four spaces.";
char* result = find_substring_with_spaces(str);
if (result) {
printf("Substring between second and third spaces: %s\n", result);
free(result); // 释放动态分配的内存
} else {
printf("No substring found.\n");
}
return 0;
}
```
这个程序会输出从第二个空格到第三个空格之间的子串(包含这两个空格),如果找不到这样的子串,则输出"No substring found."。
js截取空格后面的字符串
可以使用JavaScript的字符串方法trim()和substring()来截取空格后面的字符串,示例代码如下:
```javascript
var str = "Hello World";
var trimmed = str.trim(); // 去除字符串两端的空格
var result = trimmed.substring(trimmed.indexOf(" ") + 1); // 截取空格后面的字符串
console.log(result); // 输出 "World"
```
在上面的代码中,首先使用trim()方法去除字符串两端的空格。然后使用indexOf()方法找到第一个空格的位置。最后使用substring()方法从空格后面开始截取字符串。注意,这里的索引值要加1,否则会包含空格本身。
阅读全文
相关推荐
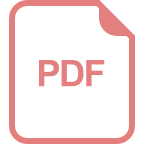
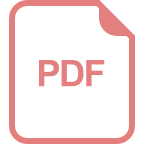
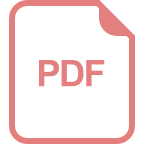













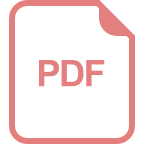