编写一个使用服务获取验证码的安卓程序,当点击该程序“获取验证码”按钮时,使用服务实现倒计时60秒的功能,并将倒计时的时间显示在“获取验证码”的按钮上
时间: 2024-12-23 07:16:28 浏览: 6
在Android中编写这样一个程序,你可以使用`CountDownTimer`类配合一个Service来实现倒计时功能。下面是一个简单的步骤说明:
1. **创建Service**:
首先,创建一个新的Service类,比如`VerificationCodeService.java`,用于处理倒计时和发送验证码的任务。这个Service会启动一个线程并运行一个CountDownTimer实例。
```java
// VerificationCodeService.java
public class VerificationCodeService extends Service {
private CountDownTimer countDownTimer;
private TextView textView;
@Override
public void onCreate() {
super.onCreate();
// 获取倒计时视图
textView = findViewById(R.id.text_view_timer);
startTimer(60); // 初始化60秒倒计时
}
private void startTimer(int seconds) {
countDownTimer = new CountDownTimer(seconds * 1000, 1000) { // 每隔1秒更新一次
@Override
public void onTick(long l) {
int remainingSeconds = (int) (seconds - (l / 1000));
textView.setText("获取验证码 (" + remainingSeconds + " 秒)");
}
@Override
public void onFinish() {
// 当倒计时结束时,停止倒计时并可能发送验证码
textView.setText("获取验证码");
stopTimer(); // 停止计时
}
}.start();
}
private void stopTimer() {
if (countDownTimer != null) {
countDownTimer.cancel();
}
}
// 其他必要的Service生命周期方法...
}
```
2. **关联按钮点击事件**:
在Activity中,你需要绑定Service并在"获取验证码"按钮的点击事件中启动Service。例如:
```java
// MainActivity.java 或其他相关的Activity
Button buttonGetCode = findViewById(R.id.button_get_code);
buttonGetCode.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
bindService(new Intent(this, VerificationCodeService.class), mConnection, BIND_AUTO_CREATE);
}
});
```
3. **创建Service连接**:
创建一个ServiceConnection实例来管理Service的生命周期:
```java
private final ServiceConnection mConnection = new ServiceConnection() {
@Override
public void onServiceConnected(ComponentName componentName, IBinder service) {
// 服务已连接,现在可以操作它
((VerificationCodeBinder) service).setTextView(textView);
}
@Override
public void onServiceDisconnected(ComponentName componentName) {
// 服务断开连接
}
};
// 使用bindService方法连接Service
Intent intent = new Intent(this, VerificationCodeService.class);
bindService(intent, mConnection, BIND_AUTO_CREATE);
```
4. **注意**: 这里假设你有一个名为`VerificationCodeBinder`的接口,用于从Service向Activity传递视图引用。这通常通过Binder机制实现,这里省略了详细部分。
在实际应用中,别忘了添加权限声明、Service的生命周期管理和错误处理。完成以上步骤后,用户点击“获取验证码”按钮,倒计时就会开始显示在按钮上。
阅读全文
相关推荐
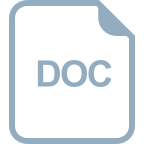
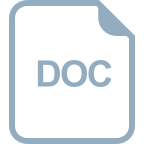
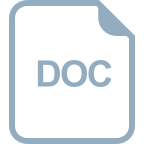









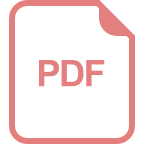
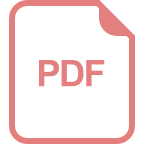
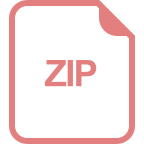
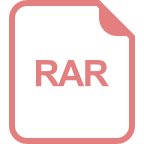
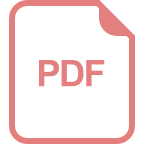
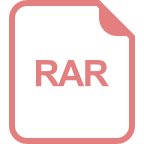
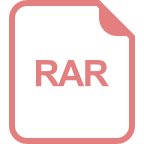