java MybatisPlus的使用
时间: 2024-10-12 19:02:47 浏览: 38
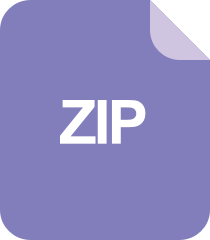
MybatisPlus代码生成器(新)
MybatisPlus是基于MyBatis的轻量级持久层框架,它简化了数据访问操作,通过注解使得映射XML文件变得不再复杂。以下是MybatisPlus的基本使用步骤:
1. **添加依赖**:在项目的pom.xml或者build.gradle文件中添加MybatisPlus的依赖。
2. **启用插件**:在启动类上加上`@MapperScan("com.example.mapper")` 注解,指定mapper接口所在的包路径。
3. **创建实体类**:实体类需要继承自`BaseEntity`,并设置主键属性为`@TableId`注解。
4. **CRUD操作**:利用Repository接口(通常是驼峰命名)进行增删改查操作,如`insert()`、`selectOneById()`等,MybatisPlus会自动完成SQL生成和结果处理。
5. **分页查询**:使用`Page`和`QueryWrapper`进行分页查询,例如`List<User> list = userMapper.selectPage(new Page<>(page, limit), new QueryWrapper<User>())`。
6. **动态条件查询**:`QueryWrapper`提供了丰富的链式API,可以方便地构建复杂的查询条件。
7. **关联查询**:支持一对一、一对多和多对多的关系映射,可通过`@TableField(exist = false)`注解避免懒加载。
阅读全文
相关推荐
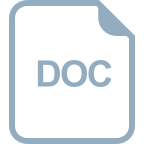
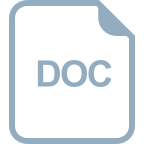

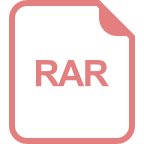
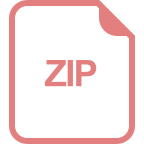
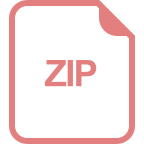










