c++代码十进制转化为n进制,n大于10用字母表示
时间: 2024-09-23 19:13:23 浏览: 51
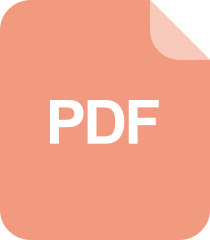
c++ 10进制转2、8、16进制.pdf
在C++中,将十进制数转换成任意基数(包括大于10的数字如十六进制或二十六进制,其中大于10的数字用字母表示)通常涉及循环、除法和取余操作。这里是一个简单的函数示例,它使用了`std::string`来构建n进制字符串:
```cpp
#include <iostream>
#include <string>
// 定义字符映射,将从10开始到9+对应的字母(A-Z)
const char digit_to_char[] = {'0', '1', '2', '3', '4', '5', '6', '7', '8', '9', 'A', 'B', 'C', 'D', 'E', 'F'};
char decimal_to_n_base(int num, int base) {
if (num == 0)
return digit_to_char[0];
// 将10以上的数字转换为对应的字母
static const int digit_limit = sizeof(digit_to_char) - 1;
while (num > digit_limit) {
num /= base;
}
return digit_to_char[num];
}
void decimal_to_string(int decimal, int base, std::string& result) {
if (decimal < 0)
throw std::invalid_argument("Negative numbers not supported");
if (base <= 1)
throw std::invalid_argument("Base must be greater than 1");
result.clear();
do {
result += decimal_to_n_base(decimal % base, base);
decimal /= base;
} while (decimal > 0);
// 添加前导零,如果需要
for (size_t i = result.size(); i < base; ++i)
result.insert(0, "0");
}
int main() {
try {
int decimal_num = 12345;
int base = 16; // 转换为十六进制
std::string n_base_str;
decimal_to_string(decimal_num, base, n_base_str);
std::cout << "Decimal " << decimal_num << " in base " << base << " is: " << n_base_str << std::endl;
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
```
这个程序首先定义了一个辅助函数`decimal_to_n_base`用于处理单个位的转换,然后在`decimal_to_string`函数中通过递归的方式从低位到高位依次获取每一位,并将其添加到结果字符串中。
阅读全文
相关推荐
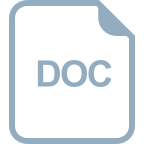
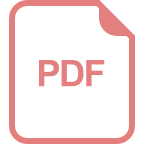
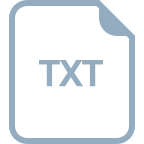
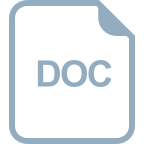
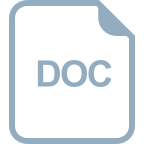
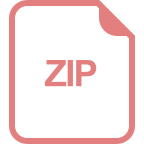
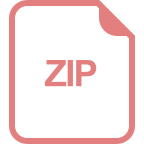
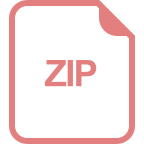
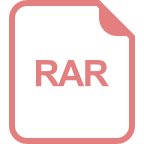
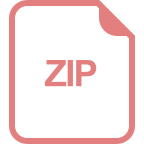
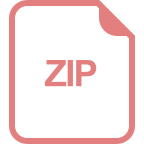
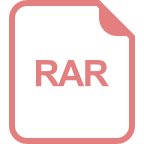
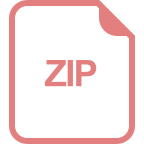