java连接mysql数据库实现插入语句
时间: 2024-09-27 13:02:05 浏览: 19
在Java中,我们可以使用JDBC (Java Database Connectivity) API来连接MySQL数据库并执行插入操作。以下是一个简单的示例,展示如何创建Connection、Statement或者PreparedStatement,并插入一条记录:
```java
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
public class MysqlInsertExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/mydatabase"; // 数据库URL
String username = "root";
String password = "yourpassword";
try {
// 加载MySQL JDBC驱动
Class.forName("com.mysql.cj.jdbc.Driver");
// 创建连接
Connection connection = DriverManager.getConnection(url, username, password);
// 插入语句
String sql = "INSERT INTO mytable (column1, column2) VALUES (?, ?)";
PreparedStatement preparedStatement = connection.prepareStatement(sql);
// 设置参数值
preparedStatement.setString(1, "value1");
preparedStatement.setInt(2, 42); // 或者其他数据类型
// 执行插入
int rowsInserted = preparedStatement.executeUpdate();
if (rowsInserted > 0) {
System.out.println("A new row was inserted successfully.");
} else {
System.out.println("Insertion failed.");
}
// 关闭资源
preparedStatement.close();
connection.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
在这个例子中,我们首先加载MySQL的JDBC驱动,然后创建一个到数据库的连接。接着,创建一个预编译的PreparedStatement来防止SQL注入攻击,并设置参数值。最后,通过`executeUpdate()`方法执行插入操作。
相关推荐
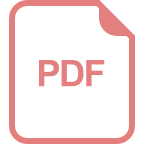
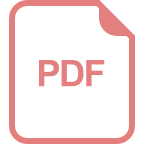
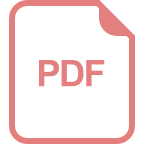














