在用visual studio设计的窗体应用中,怎么根据用户在text控件里输入的文字修改和它相连的对应的数据库中的列的值,代码是什么
时间: 2024-12-14 12:26:34 浏览: 4
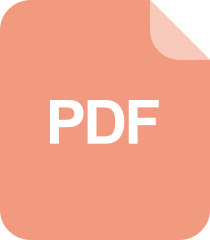
使用VisualStudio2005设计设备应用程序的用户界面和数据
在Visual Studio设计的Windows Form应用程序中,为了根据用户在`TextBox`控件中输入的内容更新数据库中的相应字段,你需要使用ADO.NET(ActiveX Data Objects for .NET)来处理数据访问。以下是一个简单的示例,展示如何使用C#编写这样的功能:
首先,确保已经添加了对`System.Data.SqlClient`命名空间的引用,并且有一个连接字符串(`ConnectionString`)用于连接到数据库。
1. 创建一个SqlConnection对象:
```csharp
string connectionString = "your_connection_string_here";
using (SqlConnection connection = new SqlConnection(connectionString))
{
```
2. 在`TextBox`的事件处理器(如TextChanged)中,获取文本框中的内容:
```csharp
private void textBox_TextChanged(object sender, EventArgs e)
{
string inputText = textBox.Text;
}
```
3. 使用SqlCommand对象来执行SQL命令(这里假设你要更新的是名为`YourTable`的表中名为`ColumnName`的列):
```csharp
private void UpdateDatabase()
{
SqlCommand command = new SqlCommand($"UPDATE YourTable SET ColumnName = @Input WHERE SomeUniqueID = 'some_unique_id'", connection);
command.Parameters.AddWithValue("@Input", inputText);
try
{
connection.Open();
int rowsAffected = command.ExecuteNonQuery();
if (rowsAffected > 0)
MessageBox.Show("Data updated successfully.");
}
catch (Exception ex)
{
MessageBox.Show("Error updating data: " + ex.Message);
}
}
```
4. 将UpdateDatabase函数与`textBox_TextChanged`关联起来,以便实时更新:
```csharp
private void textBox_TextChanged(object sender, EventArgs e)
{
UpdateDatabase();
}
```
5. 别忘了关闭SqlConnection:
```csharp
connection.Close();
}
```
阅读全文
相关推荐
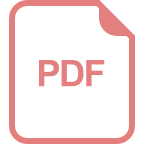
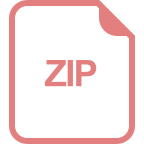
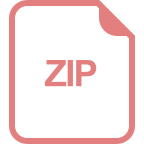
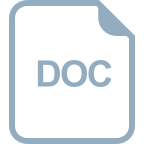
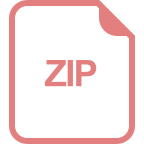
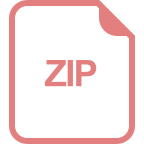
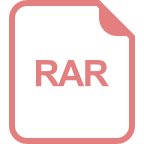
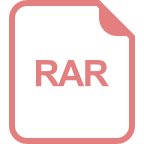
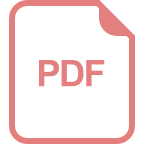
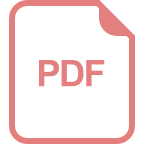
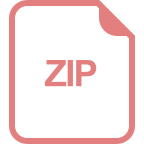
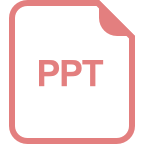
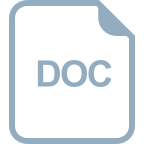
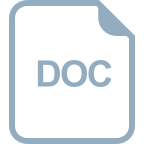
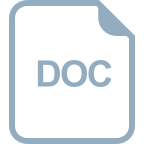