编写一个脚本,该脚本读取指定文件夹中的所有图片文件名,并与另一个Excel工作表中的一列内容进行匹配。如果有对应文件,在Excel中增加一列,相应行单元格填充绿色,表示图片存在;若没有此文件,单元格填充为黄色,表明图片缺失。请提供相应的Python或类似编程语言实现代码。
时间: 2024-12-18 08:22:56 浏览: 19
要实现这个功能,我们可以使用Python的`os`, `pandas`, 和 `openpyxl`库。首先安装必要的库(如果还没有安装):
```bash
pip install pandas openpyxl
```
然后,你可以按照以下步骤编写Python代码:
```python
import os
import pandas as pd
# 定义两个函数
def read_image_names(directory):
image_files = []
for filename in os.listdir(directory):
if filename.endswith(('.jpg', '.jpeg', '.png', '.gif')):
image_files.append(filename)
return image_files
def compare_and_fill_color(sheet, images, color_mapping):
df = sheet.to_numpy()
for index, row in enumerate(df):
file_name = row[0] # 假设Excel的第一列存储了文件名
if file_name in images:
color = color_mapping['exists']
else:
color = color_mapping['missing']
df[index][sheet.ncols - 1] = color # 添加新的列并填色
return pd.DataFrame(df)
# 读取Excel文件
excel_file = 'your_excel_file.xlsx' # 替换为实际的文件路径
workbook = pd.read_excel(excel_file)
sheet = workbook.active # 默认活动工作表
# 文件夹路径
image_dir = 'your_image_directory' # 替换为实际的文件夹路径
images = read_image_names(image_dir)
# 创建颜色映射字典
color_mapping = {'exists': 'green', 'missing': 'yellow'}
# 比较并填充颜色
new_column_index = sheet.max_column + 1 # 获取最后一列后的索引位置
sheet.insert_cols(new_column_index) # 插入新列
sheet = compare_and_fill_color(sheet, images, color_mapping)
# 保存更新后的Excel文件
workbook.save(excel_file)
```
确保替换上述代码中的`your_excel_file.xlsx`和`your_image_directory`为实际的Excel文件路径和图像文件夹路径。
阅读全文
相关推荐




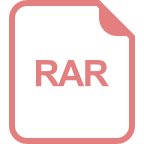













