用opencv生成4位数字验证码,数字不重叠,数字随机小角度旋转
时间: 2024-04-17 18:24:55 浏览: 139
您可以使用OpenCV库来生成4位数字验证码。以下是一个示例代码,可以生成数字不重叠且随机小角度旋转的验证码:
```python
import cv2
import numpy as np
import random
# 生成4位随机数字验证码
def generate_verification_code():
code = random.sample(range(10), 4)
return code
# 生成随机小角度旋转的验证码图像
def generate_rotated_verification_code(code):
width, height = 200, 100
font = cv2.FONT_HERSHEY_SIMPLEX
img = np.zeros((height, width, 3), np.uint8)
img.fill(255) # 白色背景
for i, digit in enumerate(code):
# 随机生成小角度旋转的角度
angle = random.uniform(-10, 10)
# 随机生成数字的位置
x = random.randint(i * width // 4, (i + 1) * width // 4 - 50)
y = random.randint(50, height - 50)
# 在图像上绘制旋转后的数字
M = cv2.getRotationMatrix2D((x, y), angle, 1)
cv2.putText(img, str(digit), (x, y), font, 2, (0, 0, 0), 3)
img = cv2.warpAffine(img, M, (width, height))
return img
# 生成验证码并显示
code = generate_verification_code()
img = generate_rotated_verification_code(code)
cv2.imshow("Verification Code", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这段代码中,`generate_verification_code` 函数用于生成4位随机数字验证码,`generate_rotated_verification_code` 函数用于生成随机小角度旋转的验证码图像。您可以根据需要调整图像的尺寸、字体样式等参数。最后,通过调用`cv2.imshow`函数显示生成的验证码图像。
请注意,为了运行以上代码,您需要确保已经安装了OpenCV库,并且保证在运行代码时有可用的显示界面。
阅读全文
相关推荐
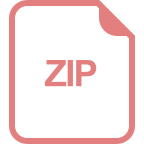

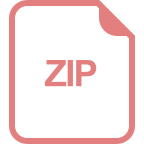
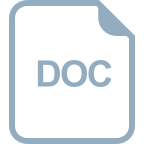


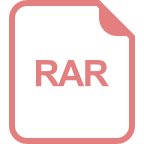
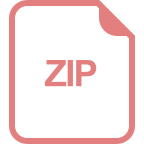
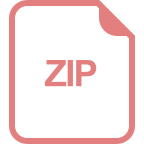
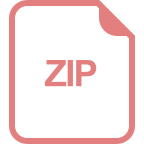
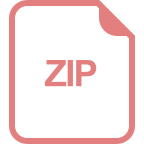
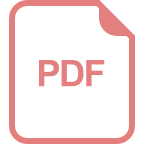
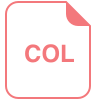
