用面向对象可视化编程C++定义一个字符串类,重载的运算符包括:=、+、+=、>、<、>=、<=、==、!=。其中,除=、+=运算符用成员函数定义外、其余的运算符要求用友元方式定义。
时间: 2024-10-21 10:05:38 浏览: 24
在C++中,为了实现面向对象可视化编程并定义一个可以重载运算符的字符串类(String),你可以创建一个名为`MyString`的类,并通过友元机制来处理除赋值和连接操作以外的比较运算符。以下是这个类的基本定义:
```cpp
#include <iostream>
#include <string>
class MyString {
public:
// 定义构造函数和析构函数
MyString(const std::string& str = "") : data(str) {}
~MyString() {}
// 成员函数实现赋值和连接操作
MyString& operator=(const MyString& other) {
if (this != &other)
data = other.data;
return *this;
}
MyString& operator+=(const MyString& other) {
data += other.data;
return *this;
}
// 友元方式实现比较运算符
friend bool operator>(const MyString& a, const MyString& b);
friend bool operator<(const MyString& a, const MyString& b);
friend bool operator>=(const MyString& a, const MyString& b);
friend bool operator<=(const MyString& a, const MyString& b);
friend bool operator==(const MyString& a, const MyString& b);
friend bool operator!=(const MyString& a, const MyString& b);
private:
std::string data; // 存储字符串的数据
};
// 定义上述友元函数的具体实现
bool operator>(const MyString& a, const MyString& b) {
return a.data > b.data;
}
// 其他运算符的实现类似,这里省略
int main() {
MyString s1("Hello");
MyString s2("World");
s1 = "C++";
s1 += "!";
std::cout << (s1 > s2 ? "s1 is greater" : "s1 is not greater") << '\n';
return 0;
}
```
阅读全文
相关推荐
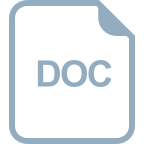
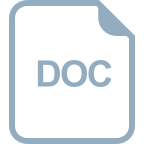
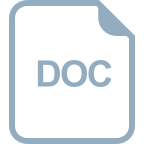
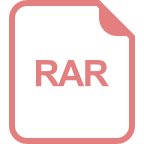
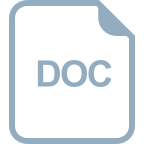
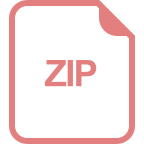
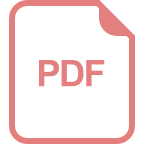
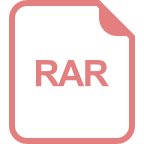
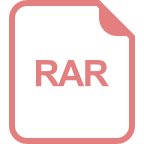
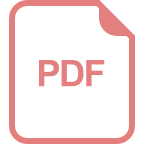
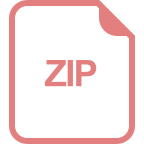
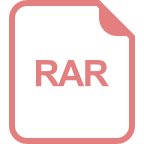
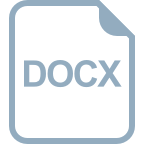
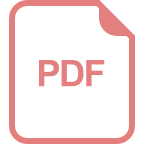
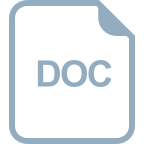
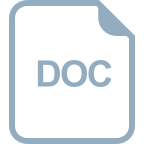
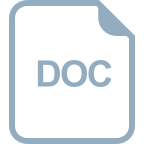
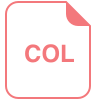
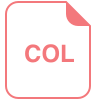