用基础的c语言方法编程。输入一个字符串,内有数字和非数字字符,如:ak123x456 17960? 302gef4563,将其中连续的数字作为一个整体,依次存放到一数组a中,例如123放入a[0],456放入a[1]...编程统计共有多少个整数,并输出这些数。
时间: 2024-10-09 08:14:22 浏览: 34
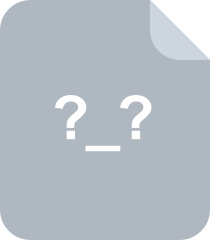
C语言数字ASCII转换成16进制互转.c

在C语言中,你可以使用`fgets`函数读取字符串,然后通过遍历字符串并判断每个字符是否为数字来实现这个需求。这里是一个简单的示例程序:
```c
#include <stdio.h>
#include <ctype.h>
#define MAX_DIGITS 100
void process_string(char* str, int arr[]) {
int count = 0;
char num[MAX_DIGITS + 1]; // 存储连续数字的临时数组
int i = 0;
while (str[i]) {
if (isdigit(str[i])) { // 如果是数字字符
num[count++] = str[i] - '0'; // 转换为数字并存储
} else if (count > 0) { // 如果遇到非数字字符并且之前有数字
arr[count] = num[0] * 10; // 将前面的数字加到当前数
for (int j = 1; j < count; ++j)
arr[count] += num[j];
printf("%d ", arr[count++]); // 输出并清零临时数组
}
i++;
}
if (count > 0) { // 处理最后一个完整数字
arr[count] = num[0] * 10; // 同上
for (int j = 1; j < count; ++j)
arr[count] += num[j];
printf("%d\n", arr[count++]);
}
}
int main() {
char input[] = "ak123x456 17960? 302gef4563";
int result[MAX_DIGITS] = {0}; // 定义结果数组
int total_count = 0;
process_string(input, result);
printf("Total integers found: %d\n", total_count);
for (int i = 0; i <= total_count; i++) {
if (result[i])
printf("Integer %d: ", i);
else
break;
}
return 0;
}
```
在这个程序中,我们首先初始化一个临时数组`num`用于存储连续的数字。当遇到非数字字符时,我们将`num`中的数字转换为整数并添加到结果数组`arr`中。最后,我们在`main`函数中输出总的整数个数以及每个整数。
阅读全文
相关推荐
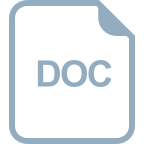
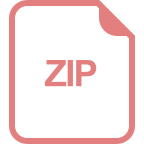
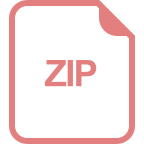
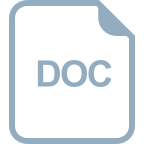
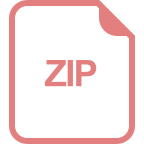
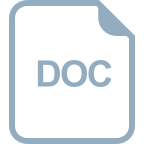
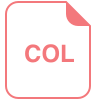

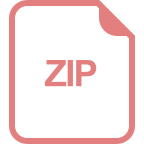
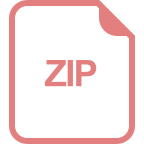
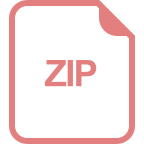