Android 读取Excel
时间: 2023-10-13 07:44:57 浏览: 25
在 Android 中读取 Excel 文件可以使用 Apache POI 库来实现。下面是一个简单的示例代码,演示如何读取 Excel 文件中的数据:
1. 首先,确保在项目的 build.gradle 文件中添加了 Apache POI 的依赖项:
```groovy
implementation 'org.apache.poi:poi:4.1.2'
implementation 'org.apache.poi:poi-ooxml:4.1.2'
```
2. 创建一个方法来读取 Excel 文件:
```java
import java.io.FileInputStream;
import java.io.IOException;
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelReader {
public static void readExcel(String filePath) {
try (FileInputStream fis = new FileInputStream(filePath);
Workbook workbook = new XSSFWorkbook(fis)) {
Sheet sheet = workbook.getSheetAt(0); // 获取第一个工作表
for (Row row : sheet) {
for (Cell cell : row) {
switch (cell.getCellType()) {
case STRING:
System.out.print(cell.getStringCellValue() + "\t");
break;
case NUMERIC:
System.out.print(cell.getNumericCellValue() + "\t");
break;
case BOOLEAN:
System.out.print(cell.getBooleanCellValue() + "\t");
break;
case BLANK:
System.out.print("\t");
break;
default:
System.out.print(cell + "\t");
}
}
System.out.println();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
3. 调用 `readExcel` 方法并传入 Excel 文件的路径:
```java
String filePath = "/path/to/your/excel.xlsx";
ExcelReader.readExcel(filePath);
```
请确保将 `filePath` 替换为您实际的 Excel 文件路径。
这是一个基本的例子,您可以根据需要进行更多的自定义和处理。
相关推荐
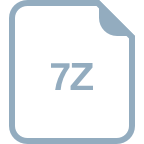
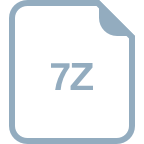














