java中进行.zip,.rar,.7z进行解压方法
时间: 2024-12-18 09:23:49 浏览: 7
在Java中,你可以使用内置的`java.util.zip`包处理`.zip`文件,而对于`.rar`和`.7z`这类更复杂的压缩格式,通常需要第三方库的支持。以下是处理`.zip`文件的基本步骤:
1. 导入所需的库:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.util.jar.JarEntry;
import java.util.jar.JarFile;
```
2. 使用`JarFile`类解压`zip`文件:
```java
try {
FileInputStream fis = new FileInputStream(new File("your_zip_file.zip"));
JarFile jar = new JarFile(fis);
Enumeration<JarEntry> entries = jar.entries();
while (entries.hasMoreElements()) {
JarEntry entry = entries.nextElement();
String name = entry.getName();
if (!entry.isDirectory()) { // 只解压文件,跳过目录
File outFile = new File("output_directory", name);
jarEntry.extractToPath(outFile.getParent(), true); // 解压到指定目录
}
}
jar.close();
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
```
对于`.rar`和`.7z`,你需要使用像`org.apache.commons.compress`这样的第三方库。例如,`7z`支持可以使用`net.sf.jopt-simple`作为命令行解析库。
安装并导入这些库后,你可以通过创建`Executors`来执行外部命令,如7-Zip或RAR工具。这里只是一个大概的概念,实际操作可能涉及到更复杂的安全性和错误处理。
```java
import org.apache.commons.compress.utils.IOUtils;
import net.sf.jopt-simple.OptionParser;
public void extractRarOr7z(String file, String targetDir) {
try {
ProcessBuilder pb = new ProcessBuilder("your_rar_or_7z_command", "-o", targetDir, file);
Process process = pb.start();
int exitCode = process.waitFor();
IOUtils.copyLarge(process.getInputStream(), System.out);
if (exitCode != 0) {
throw new RuntimeException("Error executing command");
}
} catch (Exception e) {
e.printStackTrace();
}
}
```
阅读全文
相关推荐
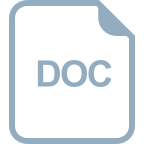
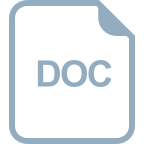
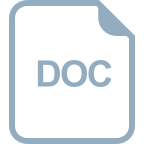
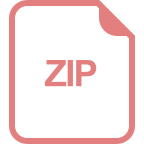
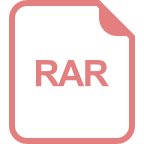
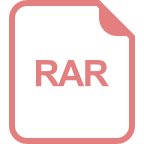
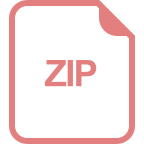
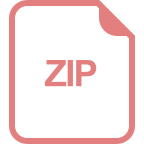
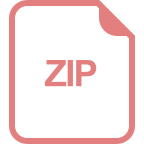
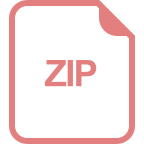


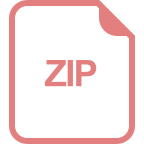
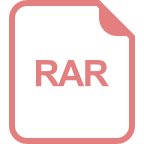
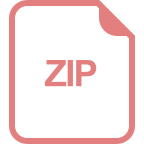
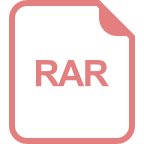
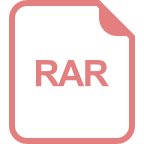
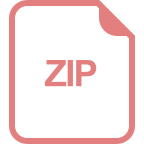