A*算法求解八数码问题代码python
时间: 2024-11-19 09:10:14 浏览: 9
A*算法是一种启发式搜索算法,常用于路径规划,包括解决八皇后问题这样的回溯问题。在Python中实现A*算法来解决八数码问题,首先需要明确目标状态、起点和启发函数。以下是简化的A*算法伪代码:
```python
import heapq
def a_star_search(board, start, goal):
frontier = [(0, start)] # 开放列表,(f_score, state)
came_from = {}
cost_so_far = {}
def heuristic(state): # 启发函数,计算从当前状态到目标状态的代价估计
row, col = state
return max(abs(row - goal[0]), abs(col - goal[1]))
while frontier:
current = heapq.heappop(frontier)[1] # 取出f_score最小的节点
if current == goal: # 找到了目标
break
for neighbor in get_neighbors(board, current): # 获取相邻未访问节点
tentative_g_score = cost_so_far[current] + 1 # 距离加一
if neighbor not in cost_so_far or tentative_g_score < cost_so_far[neighbor]:
cost_so_far[neighbor] = tentative_g_score
priority = tentative_g_score + heuristic(neighbor) # f_score = g_score + h_score
heapq.heappush(frontier, (priority, neighbor))
came_from[neighbor] = current
solution_path = []
current = goal
while current != start:
solution_path.append(current)
current = came_from[current]
solution_path.append(start) # 添加起始位置
solution_path.reverse() # 逆序得到实际路径
return solution_path
# 辅助函数:获取某个位置的邻居
def get_neighbors(board, pos):
row, col = pos
neighbors = [(row+1, col), (row-1, col), (row, col+1), (row, col-1)]
valid_neighbors = [n for n in neighbors if 0 <= n[0] < len(board) and 0 <= n[1] < len(board[0]) and board[n] == 0]
return valid_neighbors
# 示例用法
board = [[5,3,0,0,7,0,0,0,0],[6,0,0,1,9,5,0,0,0],[0,9,8,0,0,0,0,6,0],[8,0,0,0,6,0,0,0,3],[4,0,0,8,0,3,0,0,1],[7,0,0,0,2,0,0,0,6],[0,6,0,0,0,0,2,8,0],[0,0,0,4,1,9,0,0,5],[0,0,0,0,8,0,0,7,9]]
start = (0, 0)
goal = (len(board)-1, len(board[0])-1)
path = a_star_search(board, start, goal)
```
阅读全文
相关推荐
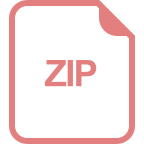
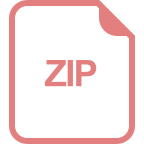















