LocalDateTime之间的时长
时间: 2024-11-01 07:06:12 浏览: 6
LocalDateTime类表示日期和时间信息,但它本身并不包含两个时刻之间的时间差。如果你想要计算两个LocalDateTime对象之间的时长,你可以先将它们转换为Duration类。以下是步骤:
1. 获取两个LocalDateTime实例。
2. 使用java.time.temporal.TemporalUnit枚举类型(如Days, Hours, Minutes等)指定单位。
3. 调用`Duration.between()`方法,传入这两个LocalDateTime对象作为参数。
例如:
```java
LocalDateTime start = LocalDateTime.of(2023, Month.JANUARY, 1, 0, 0);
LocalDateTime end = LocalDateTime.of(2023, Month.JANUARY, 15, 12, 30);
Duration duration = Duration.between(start, end);
long totalSeconds = duration.toSeconds();
```
`totalSeconds`现在就包含了两个LocalDateTime之间的总秒数。如果你想得到其他单位(如小时、分钟),可以继续转换。
相关问题
计算两个LocalDateTime的相差时长
在Java中,你可以使用`Duration`类来计算两个`LocalDateTime`对象之间的相隔时间。首先,你需要将这两个日期时间转换到`ZonedDateTime`对象,这是因为`Duration`是基于时间点的时间间隔,而`LocalDateTime`并不包含时区信息。
以下是一个示例:
```java
import java.time.Duration;
import java.time.LocalDateTime;
import java.time.ZoneId;
// 获取当前本地时间和另一个日期时间
LocalDateTime now = LocalDateTime.now();
LocalDateTime otherDateTime = LocalDateTime.of(2023, 3, 24, 12, 0); // 举例
// 将LocalDateTime转换为包含时区的ZonedDateTime
ZonedDateTime nowWithZone = now.atZone(ZoneId.systemDefault());
ZonedDateTime otherDateTimeWithZone = otherDateTime.atZone(ZoneId.systemDefault());
// 使用Duration计算两个ZonedDateTime的时间差
Duration timeDifference = Duration.between(nowWithZone, otherDateTimeWithZone);
// 打印结果
long seconds = timeDifference.getSeconds(); // 返回秒数
System.out.println("相差时间为:" + timeDifference.toDays() + "天" + timeDifference.getHours() + "小时"
+ timeDifference.getMinutes() + "分钟" + timeDifference.getSeconds() + "秒");
```
在这个例子中,`Duration.between()`方法会返回一个`Duration`对象,表示两个时间点之间的时间间隔。
有两个 LocalDateTime 格式的数据,差天了计算出 HH:mm:ss格式的时长,使用java代码生成出来
在 Java 中,你可以通过 `Duration` 类或者自定义逻辑来计算两个 `LocalDateTime` 对象之间的时长,并转换为 `HH:mm:ss` 格式。以下是一个示例:
```java
import java.time.LocalDateTime;
import java.time.Duration;
import java.time.format.DateTimeFormatter;
public class Main {
public static void main(String[] args) {
// 假设你有这两个 LocalDateTime 对象
LocalDateTime start = LocalDateTime.of(2023, 4, 1, 10, 0, 0);
LocalDateTime end = LocalDateTime.of(2023, 4, 2, 15, 30, 0);
// 计算时长
Duration duration = Duration.between(start, end);
// 将 Duration 转换为小时、分钟和秒
int hours = duration.toHours();
int minutes = duration.toMinutes() % 60; // 取余操作得到分钟数
int seconds = duration.getSeconds();
// 格式化成 "HH:mm:ss" 的字符串
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("HH:mm:ss");
String formattedDuration = String.format("%02d:%02d:%02d", hours, minutes, seconds);
System.out.println("时长:" + formattedDuration);
}
}
```
在这个例子中,我们首先获取了两个日期时间点之间的差异,然后将 `Duration` 转换为小时、分钟和秒。最后,使用 `DateTimeFormatter` 格式化输出结果。
阅读全文
相关推荐
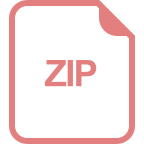
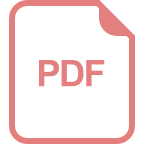
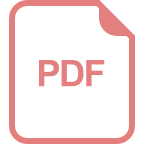
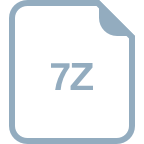
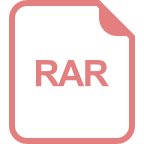
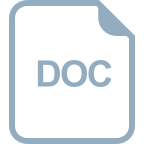
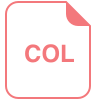
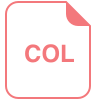







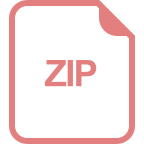
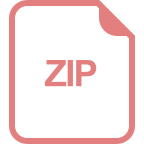