c语言求最接近的价格
时间: 2024-09-13 17:02:26 浏览: 37
在C语言中,如果你需要找出一组价格中最接近某个特定价格的值,你可以通过遍历数组并比较每个元素与目标值之间的差来实现。这里是一个简单的示例:
```c
#include <stdio.h>
#include <math.h> // 包含fabs函数来计算绝对值
// 定义结构体表示价格和索引
typedef struct {
double price;
int index; // 用于记录价格在原数组中的位置
} Price;
// 比较两个价格,返回更接近目标价格的那个
Price compare_price(Price a, Price b, double target) {
double diff_a = fabs(a.price - target);
double diff_b = fabs(b.price - target);
if (diff_a < diff_b)
return a;
else
return b;
}
// 主函数
int main() {
double prices[] = {10.5, 25.8, 19.99, 30.4, 22.7};
double target_price = 25.0;
int num_prices = sizeof(prices) / sizeof(prices[0]);
Price closest_price = prices[0];
for (int i = 1; i < num_prices; i++) {
closest_price = compare_price(closest_price, prices[i], target_price);
}
printf("最接近 %f 的价格是 %.2lf,它的索引是 %d\n", target_price, closest_price.price, closest_price.index);
return 0;
}
```
在这个程序中,我们首先定义了一个`compare_price`函数,它接受两个价格以及一个目标价格,并根据它们与目标价格的差距选择更接近的一个。然后在主函数中,我们遍历价格数组,每次迭代都将找到的当前最接近的价格与下一个价格进行比较。
阅读全文
相关推荐
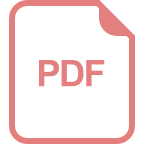
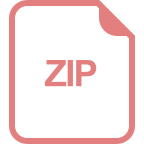
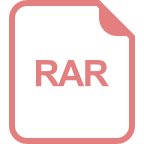
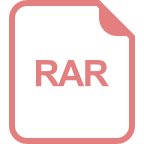
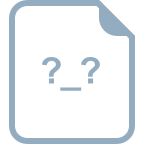
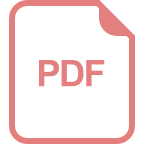
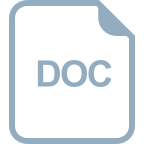
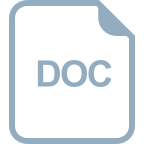
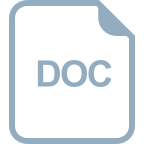
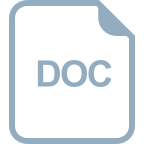
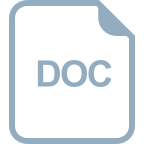
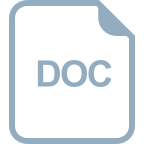
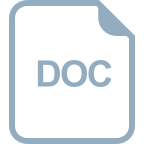
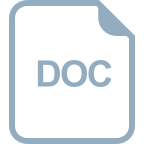
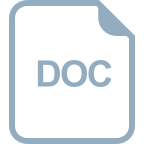
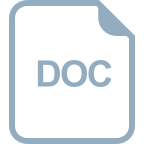
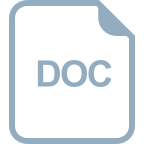
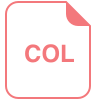