使用Qt C++ 帮我实现一个保存后缀是“.prc"文件的功能,点击保存按钮,给出一个弹窗,弹出固定文件夹路径,可以选该文件中的文件夹或新建一层文件夹(仅能新建一层文件夹), 打开要保存的文件夹,保存文件到该文件夹中,或者选择已存在的文件,弹出是否覆盖提示框,给出详细的demo
时间: 2024-12-16 08:14:08 浏览: 3
在Qt中,实现这样的功能需要使用QFileDialog(文件对话框)、QStandardPaths(标准路径管理)以及QSaveFile(保存文件)等。下面是一个简单的示例代码:
```cpp
#include <QApplication>
#include <QFileDialog>
#include <QStandardPaths>
#include <QSaveFile>
// 省略窗口头文件和UI设置...
void on_saveButton_clicked() {
// 获取默认的文档保存路径
QString savePath = QStandardPaths::writableLocation(QStandardPaths::DocumentsLocation);
// 弹出文件对话框,让用户选择保存位置
QString selectedPath;
QStringList filter {"*.prc"};
if (selectedPath.isEmpty()) {
QFileDialog dialog(this);
dialog.setNameFilter(filter.join(";;"));
dialog.setAcceptMode(QFileDialog::AcceptSave);
dialog.setDirectory(savePath);
if (dialog.exec()) {
selectedPath = dialog.selectedFiles().first();
}
}
// 检查用户是否选择了文件夹还是文件
if (!selectedPath.endsWith("/")) {
QMessageBox::information(this, "信息", "请选择一个文件夹以便保存文件.");
return;
}
// 创建保存文件实例,并尝试保存
QSaveFile saveFile(selectedPath + "/newfile.prc");
if (!saveFile.open(QIODevice::WriteOnly)) {
QMessageBox::critical(this, "错误", "无法打开文件进行保存: " + saveFile.errorString());
return;
}
// 判断文件是否存在,如果存在则显示覆盖提示
if (saveFile.fileName().endsWith(".prc") && saveFile.exists()) {
int reply = QMessageBox::question(this, "覆盖确认", "文件已存在,是否覆盖?", QMessageBox::Yes|QMessageBox::No, QMessageBox::No);
if (reply != QMessageBox::Yes) {
return;
}
}
// 尝试写入文件
QTextStream out(&saveFile);
out << "Content to be saved in .prc file"; // 这里替换实际的数据
// 关闭文件
saveFile.close();
QMessageBox::information(this, "成功", "文件已保存.");
}
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
YourWindow window; // 替换YourWindow为你的窗口类名
window.show();
return app.exec();
}
```
在这个例子中,首先获取用户的默认文档存储路径,然后打开文件对话框让用户选择。如果用户选择的是文件,我们会提醒他们选择文件夹。接着,我们创建一个`QSaveFile`实例尝试保存到选定的目录中。如果文件已经存在,会询问用户是否覆盖。最后,我们使用`QTextStream`将数据写入文件并关闭。
请注意,这只是一个基础的示例,实际应用中你可能需要处理更多异常情况,并且在实际项目中,你需要自定义错误处理和数据写入逻辑。
阅读全文
相关推荐
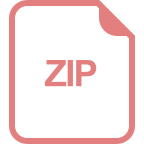
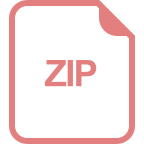
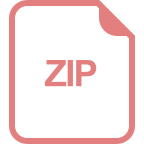
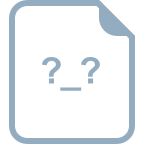
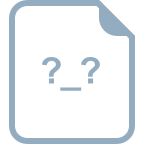
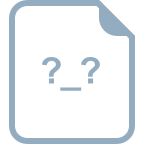
