python访问多个文件夹
时间: 2024-10-21 07:02:09 浏览: 32
在Python中,如果你需要访问多个文件夹,通常会用到os模块中的os.walk()函数,它能遍历指定目录及其所有子目录。以下是一个基本示例:
```python
import os
def traverse_folders(start_dir):
for root, dirs, files in os.walk(start_dir):
# root - 当前路径
# dirs - 目录列表
# files - 文件列表
for directory in dirs:
print(f"Directory found: {os.path.join(root, directory)}")
for file in files:
print(f"File found: {os.path.join(root, file)}")
# 要访问的起始目录
base_path = "path_to_your_directories"
traverse_folders(base_path)
```
在这个例子中,`os.walk()`生成器会递归地遍历指定的起始目录`start_dir`及其所有子目录。对于每个找到的目录和文件,都打印出它们的完整路径。
相关问题
python中同时访问多个文本文件
在Python中,你可以使用内置的`open()`函数以及`with`语句来同时访问多个文本文件。`with`语句可以确保文件在使用完毕后会被正确关闭,即使在处理过程中发生异常也是如此。
下面是一个简单的例子,展示了如何遍历一个目录并逐个打开文本文件:
```python
import os
# 指定文件夹路径
folder_path = 'path_to_your_folder'
# 遍历文件夹中的所有.txt文件
for filename in os.listdir(folder_path):
if filename.endswith('.txt'): # 确保是文本文件
file_path = os.path.join(folder_path, filename)
with open(file_path, 'r') as f:
content = f.read() # 读取文件内容
# 这里可以对每个文件的内容进行操作,例如打印、分析等
print(f"Reading from {file_path}:")
print(content)
#
阅读全文
相关推荐
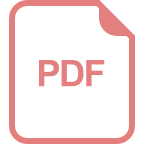
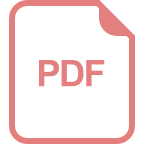
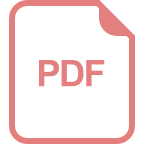
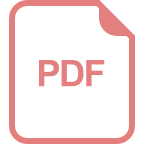
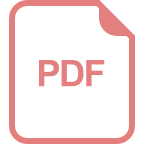
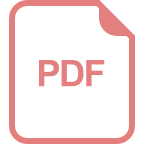
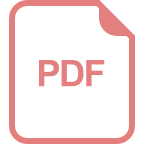
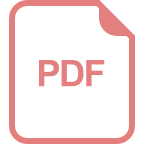
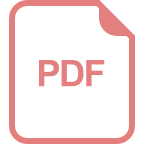
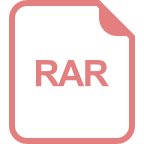
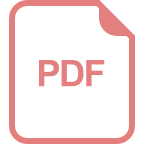
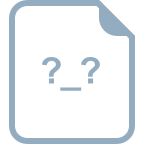
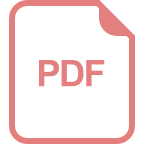
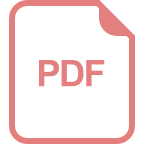
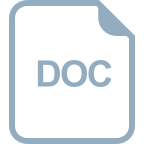
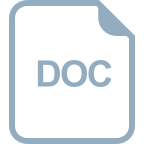
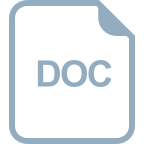