用C++给定两个字符串X和Y,编写算法寻找字符串X的最长前缀同时是字符串Y的后缀。
时间: 2024-09-27 14:08:51 浏览: 66
要解决这个问题,可以使用Knuth-Morris-Pratt (KMP) 算法[^1]来找到字符串 X 的最长前缀,同时也是字符串 Y 的后缀。KMP 算法用于高效地在主串中查找模式串的位置,即使模式串有重复字符。
这里是一个简单的 C++ 示例,展示了如何使用 KMP 算法:
```cpp
#include <iostream>
#include "kmp_algorithm.h" // 假设包含了KMP算法头文件
// 定义 KMP 函数,用于计算 pattern(Y)的失配表
void compute_lps(int pattern[], int n) {
int len = 0;
pattern[len] = -1;
for (int i = 1; i < n; ++i) {
if (pattern[i] == pattern[len]) {
len++;
pattern[i] = len;
} else {
if (len != 0)
len = pattern[len - 1];
else
pattern[i] = -1;
}
}
}
// 使用 KMP 算法查找最长公共前缀
std::string longest_common_prefix(const std::string& X, const std::string& Y) {
int m = X.size();
int n = Y.size();
// 计算 Y 的失配表
int lps[n + 1];
compute_lps(lps, n);
int i = 0, j = 0;
std::string result;
while (i < m && j < n) {
if (X[i] == Y[j])
i++, j++;
else if (j != 0)
j = lps[j - 1];
else
break;
}
return X.substr(0, i);
}
int main() {
std::string X = "ABABC";
std::string Y = "BABCA";
std::cout << "Longest common prefix of X and Y is: " << longest_common_prefix(X, Y) << std::endl;
return 0;
}
```
在这个示例中,`longest_common_prefix` 函数首先计算字符串 Y 的失配表 `lps`,然后使用 KMP 算法在 X 中查找 Y 的最长后缀。当遇到不匹配的字符时,它会利用失配表快速回溯。
阅读全文
相关推荐
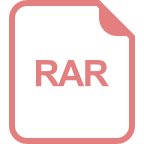
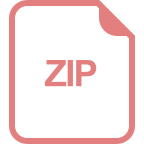
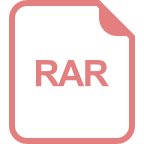











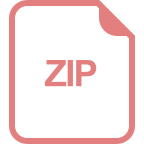
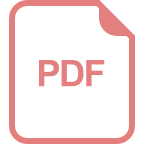
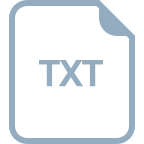
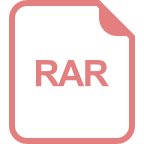
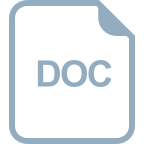