使用C语言实现1、输入线性表长度,并依次输入线性表的每一项。2、展示线性表中的内容。3、输入在第i个位置插入元素,输入元素值。4、展示线性表中的内容。5、输入在第i个位置删除元素,输入元素值。6、展示线性表中的内容
时间: 2024-09-18 19:14:24 浏览: 42
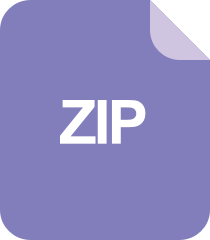
C语言实现的顺序线性表
在C语言中,我们可以使用数组或链表来实现这个功能。这里以动态链表为例,因为它的插入和删除操作更方便。
首先,我们需要定义一个结构体表示链表节点:
```c
typedef struct Node {
int data;
struct Node* next;
} Node;
```
接下来,编写函数实现上述操作:
1. 输入线性表长度和内容:
```c
void inputList(Node** head, int length) {
printf("请输入线性表长度:");
scanf("%d", &length);
*head = (Node*)malloc(sizeof(Node)); // 创建首节点
(*head)->data = 0; // 初始化第一个元素为0
(*head)->next = NULL;
for (int i = 1; i < length; i++) {
Node* newNode = (Node*)malloc(sizeof(Node));
printf("请输入第%d个元素:", i + 1);
scanf("%d", &newNode->data);
newNode->next = *head;
*head = newNode;
}
}
```
2. 展示线性表内容:
```c
void displayList(Node* head) {
Node* temp = head;
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
printf("\n");
}
```
对于插入和删除操作,可以添加类似的功能:
3. 插入元素:
```c
void insertElement(Node** head, int position, int value) {
if (position <= 0 || position > listLength(*head)) {
printf("无效的位置。\n");
return;
}
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = *head;
Node* current = *head;
for (int i = 0; i < position - 1; i++) {
current = current->next;
}
newNode->next = current->next;
current->next = newNode;
}
// 更新listLength函数,计算实际长度
int listLength(Node* head) {
int count = 0;
Node* temp = head;
while (temp != NULL) {
count++;
temp = temp->next;
}
return count;
}
```
4. 删除元素:
```c
void deleteElement(Node** head, int position, int value) {
if (position <= 0 || position > listLength(*head)) {
printf("无效的位置。\n");
return;
}
Node* current = *head;
for (int i = 0; i < position - 1; i++) {
current = current->next;
}
if (current->next == NULL || current->next->data != value) {
printf("找不到要删除的元素。\n");
return;
}
Node* temp = current->next->next;
free(current->next);
current->next = temp;
}
// 更新displayList函数,显示修改后的列表
void displayListAfterDeletion(Node* head) {
displayList(head);
}
```
5. 调用所有函数并展示最终结果:
```c
int main() {
int length;
Node* head = NULL;
// 其他步骤...
return 0;
}
```
阅读全文
相关推荐
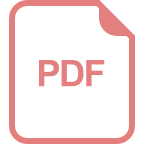


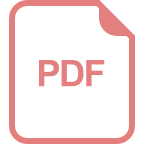
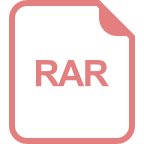
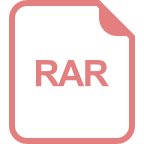
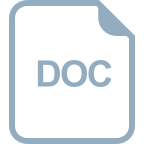
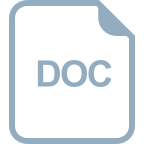
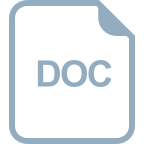
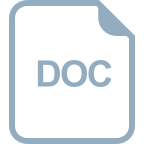
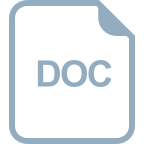
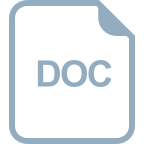



