直接给我java代码
时间: 2024-12-20 18:25:19 浏览: 2
以下是Java代码,用于解决上述寻宝游戏的路径探索问题。这里我们使用深度优先搜索(DFS)来遍历所有可能的状态,因为寻找所有可能的位置实际上是在穷举所有路径。
```java
import java.util.*;
class TreasureHunt {
static final int DIRECTIONS = 4; // 北、东、南、西
static final int[][] moves = {{0, 1}, {1, 0}, {0, -1}, {-1, 0}}; // 方向变换矩阵
public static int uniqueFinalPositions(int n, String steps) {
boolean[][] visited = new boolean[n + 1][DIRECTIONS]; // 记录已经访问过的状态
visited[0][0] = true; // 开始位置
for (int i = 0; i < n; i++) {
char step = steps.charAt(i);
if (step == 'F') {
dfs(n, i, moves[0], visited); // 前进
} else if (step == 'L') {
dfs(n, i, moves[2], visited); // 向左
} else {
dfs(n, i, moves[1], visited); // 向右
}
}
return Arrays.stream(moves)
.mapToObj(dir -> visited[n][dir])
.filter(value -> value)
.count(); // 返回最终位置的种类
}
private static void dfs(int n, int startStep, int[] dir, boolean[][] visited) {
int currentStep = startStep;
while (currentStep < n) {
visited[currentStep + 1][dir[0]] |= visited[currentStep][dir[1]];
visited[currentStep + 1][dir[1]] |= visited[currentStep][dir[2]];
visited[currentStep + 1][dir[2]] |= visited[currentStep][dir[3]];
visited[currentStep + 1][dir[3]] |= visited[currentStep][dir[0]];
dir = nextMove(dir); // 更新方向
currentStep++;
}
}
private static int[] nextMove(int[] dir) {
return dir[(dir[0] + 1) % DIRECTIONS];
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int n = scanner.nextInt();
String steps = scanner.next();
System.out.println(uniqueFinalPositions(n, steps));
}
}
```
这段代码首先定义了一个二维布尔数组 `visited` 来跟踪每个位置和方向是否已被访问过。然后,它通过一个循环模拟每一步操作,使用深度优先搜索(dfs)函数递归地尝试所有可能的变化。最后,在main方法中读取输入并调用 `uniqueFinalPositions` 函数输出结果。
阅读全文
相关推荐
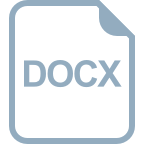
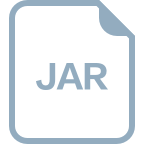
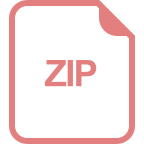
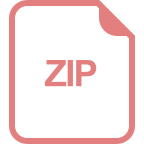
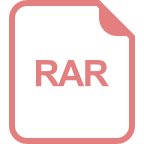
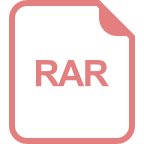
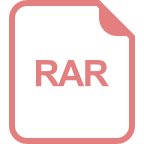
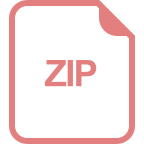
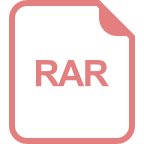
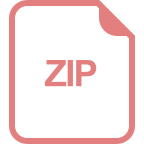
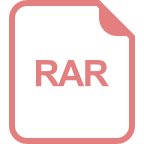