fstream 打印
时间: 2023-08-16 20:10:35 浏览: 108
您可以使用 `fstream` 类来打开文件,并将内容打印到控制台上。下面是一个使用 `fstream` 打印文件内容的示例代码:
```cpp
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream file("example.txt"); // 打开文件 example.txt
std::string line;
if (file.is_open()) {
while (std::getline(file, line)) { // 逐行读取文件内容
std::cout << line << std::endl; // 打印每行内容
}
file.close(); // 关闭文件
} else {
std::cout << "无法打开文件" << std::endl;
}
return 0;
}
```
在上述代码中,我们使用 `ifstream` 类来打开文件 `example.txt`。然后,我们使用 `std::getline` 函数逐行读取文件内容,并将每行内容打印到控制台上。最后,我们关闭文件。
请确保将代码中的 `"example.txt"` 替换为您想要打印的实际文件路径。
相关问题
c++ fstream
C++中的fstream库提供了一种用于文件输入输出的机制,它结合了ifstream和ofstream两个类的功能。使用fstream库的优点是可以同时读取和写入文件,而不必创建不同的对象来执行这些操作。
以下是使用fstream库进行文件读写的示例代码:
```c++
#include <fstream>
#include <iostream>
using namespace std;
int main() {
// 打开文件
fstream file("example.txt", ios::in | ios::out);
// 写入数据
if (file.is_open()) {
file << "Hello, World!\n";
file << "This is an example file.\n";
file.close();
} else {
cout << "Error opening file.\n";
return 1;
}
// 读取数据
string line;
file.open("example.txt", ios::in);
if (file.is_open()) {
while (getline(file, line)) {
cout << line << '\n';
}
file.close();
} else {
cout << "Error opening file.\n";
return 1;
}
return 0;
}
```
在上面的代码中,我们首先使用fstream打开一个名为"example.txt"的文件,然后使用文件流插入运算符(<<)写入数据。接着,我们重新打开文件,使用getline函数读取每一行数据,并将其打印到控制台上。
需要注意的是,fstream对象可以以不同的模式打开文件,例如:
- ios::in:打开文件进行读取。
- ios::out:打开文件进行写入。
- ios::app:在文件末尾追加数据。
- ios::binary:以二进制模式打开文件。
可以将这些模式组合使用,例如:ios::in | ios::binary。
fstream头文件
### 关于 C++ 中 `fstream` 头文件的使用说明
#### 文件流类概述
在 C++ 中,`<fstream>` 是用于处理文件输入输出的标准库头文件。此头文件定义了三个主要的类来管理文件操作:`ifstream`, `ofstream`, 和 `fstream`[^1]。
#### 类功能介绍
- **`ifstream`**: 继承自 `istream`,专门用来读取文件中的数据。
- **`ofstream`**: 继承自 `ostream`,主要用于向文件写入数据。
- **`fstream`**: 同时继承自 `iostream` 的两个基类 (`istream` 和 `ostream`) ,可以实现既可读又可写的双向文件访问[^2].
#### 基本用法实例
为了展示如何利用这些类来进行基本的操作,下面给出一段简单的代码片段:
```cpp
#include <iostream>
#include <fstream>
using namespace std;
int main() {
// 创建并打开一个文本文件进行写入
ofstream outFile;
outFile.open("example.txt");
if (!outFile.is_open()) { cout << "Failed to open file"; return 1; }
// 写一些字符串到文件里
outFile << "This is an example of using fstream.";
// 记得关闭文件
outFile.close();
// 打开刚才创建的文件准备读取内容
ifstream inFile;
string line;
inFile.open("example.txt");
while(getline(inFile, line)) {
cout << line << endl;
}
// 不再需要的时候记得关闭文件
inFile.close();
}
```
这段程序首先通过 `ofstream` 对象将一句话写入名为 `"example.txt"` 的新文件中;接着它再次打开同一个文件但是这次是以只读模式(`ifstream`) 来打印出之前存储的内容[^3].
#### 错误预防提示
当遇到像 `error C2065: 'ifstream' : undeclared identifier` 这样的编译错误时,通常是因为忘记包含必要的命名空间声明或是虽然包含了 `<fstream>` 却未引入标准库名称空间 `std::`. 正确的做法是在源码顶部加上 `using namespace std;` 或者每次调用函数前都显式指定其所属的空间如 `std::ifstream`[^4].
阅读全文
相关推荐


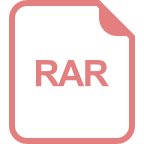
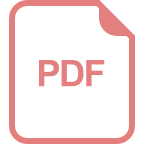










