用c++,分别验证StrCompare(S,T)和SubString(&Sub,S,pos,len)和Index(S,T,pos)和StrInsert(&S,pop,T)和StrDelete(&S,pos,len)五个函数的正确性
时间: 2024-10-21 08:17:35 浏览: 43
在 C++ 中,验证这些字符串处理函数(StrCompare、SubString、Index、StrInsert 和 StrDelete)的正确性通常需要对它们的输入和预期输出进行测试。这里我会简要说明每个函数的作用以及一种可能的验证方法:
1. **StrCompare(S, T)**: 这个函数可能是用于比较两个字符串是否相等。你可以通过提供一些相同和不同的字符串作为输入,检查返回结果是否符合预期,即两个相同字符串返回0,不同字符串返回非0值。
```cpp
// 示例测试
bool test_strcmp(const std::string &s1, const std::string &s2) {
int result = StrCompare(s1, s2);
return (s1 == s2) ? result == 0 : result != 0;
}
```
2. **SubString(&Sub, S, pos, len)**: 这个函数应该是从`S`字符串中提取子串并复制到`Sub`中。可以验证给定位置和长度的情况下,`Sub`是否等于正确的子串。
```cpp
// 测试子串提取
std::string test_substring(const std::string &s, size_t pos, size_t len, const std::string &expected_sub) {
SubString(Sub, s, pos, len);
return Sub == expected_sub;
}
```
3. **Index(S, T, pos)**: 如果这个函数查找子串`T`在`S`中的起始索引,你需要确保它在正确的位置找到或返回合理的结果(如没有找到则返回某个特定值或异常)。
```cpp
// 测试子串查找
size_t test_index(const std::string &s, const std::string &t, size_t pos, size_t expected_index) {
size_t found_pos = Index(s, t, pos);
if (found_pos != npos && found_pos >= pos)
return found_pos - pos; // 减去偏移是因为索引是从0开始的
else
return found_pos == npos ? "Not Found" : found_pos;
}
```
4. **StrInsert(&S, pop, T)**: 将子串`T`插入到`S`指定的位置`pop`。同样,检查插入后的字符串是否正确。
```cpp
// 测试插入操作
void test_insert(std::string &s, size_t pop, const std::string &t, const std::string &expected_result) {
StrInsert(s, pop, t);
if (s.substr(pop, t.length()) == t)
return;
throw std::runtime_error("Insertion failed");
}
```
5. **StrDelete(&S, pos, len)**: 删除`S`中从位置`pos`开始的`len`个字符。检查删除后的内容。
```cpp
// 测试删除操作
void test_delete(std::string &s, size_t pos, size_t len, const std::string &expected_result) {
StrDelete(s, pos, len);
if (s.substr(0, pos) + s.substr(pos+len, s.length()-pos-len) == expected_result)
return;
throw std::runtime_error("Deletion failed");
}
```
每个函数都需要编写一组针对各种情况(包括正常、边界、异常)的测试用例,并确保所有功能在所有这些条件下都能按预期工作。
阅读全文
相关推荐
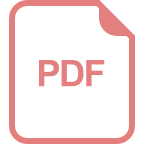
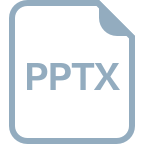
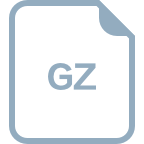





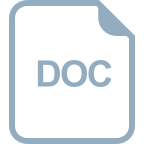









