输入一行字符(少于300个字符),以回车结束,统计其中单词的个数。各单词之间用空格分隔,空格数可以是多个。
时间: 2023-12-21 18:32:07 浏览: 35
以下是一个统计单词个数的Python程序示例:
```python
sentence = input("请输入一行字符:")
words = sentence.split()
word_count = len(words)
print("单词个数为:", word_count)
```
这个程序首先通过`input()`函数获取用户输入的一行字符,并将其赋值给变量`sentence`。然后,使用`split()`方法将`sentence`按照空格分割成一个单词列表`words`。最后,使用`len()`函数获取`words`列表的长度,即单词个数,并将结果打印出来。
相关问题
输入一行字符,统计其中单词的个数。各单词之间用空格分隔,空格数可以是多个。
可以使用字符串的split()方法将输入的一行字符按照空格分隔成一个单词列表,然后再统计列表中单词的个数即可。具体代码如下:
```python
s = input("请输入一行字符:")
words = s.split() # 按照空格分隔成单词列表
count = len(words) # 统计单词个数
print("单词个数为:", count)
```
例如,输入字符串"Hello world! I'm a Python programmer.",输出结果为:
```
单词个数为: 7
```
10. 统计单词 输入一行字符,以回车结束,统计其中单词的个数。各单词之间用空格分隔,空格数可以是多个。 用C++实现
以下是使用 C++ 实现统计单词个数的代码示例:
```cpp
#include <iostream>
#include <string>
#include <sstream>
using namespace std;
int countWords(string input) {
int count = 0;
stringstream ss(input);
string word;
while (ss >> word) {
count++;
}
return count;
}
int main() {
string input;
cout << "请输入一行字符:";
getline(cin, input);
int wordCount = countWords(input);
cout << "单词个数为:" << wordCount << endl;
return 0;
}
```
以上代码会提示用户输入一行字符,然后通过调用 `countWords()` 函数统计其中的单词个数。该函数使用 `std::stringstream` 来分割输入的字符串,并通过循环逐个读取单词,最后返回单词个数。注意,该代码假设单词之间用空格分隔。
相关推荐
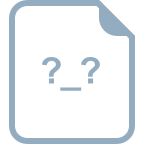
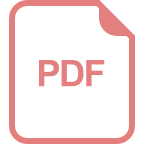












