将两个递增的有序链表合并为一个递增的有序链表。要求结果链表仍使用原来两个链表向存储空间,不另外占用其他的存储空间。表中不允许有重复的数据。用c语言编写
时间: 2024-09-22 18:07:58 浏览: 69
在C语言中,将两个递增的有序链表合并成一个递增的有序链表,可以采用迭代或递归的方式。这里我提供一个迭代的方法,因为迭代通常更节省空间。
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
typedef struct ListNode {
int val; // 节点值
struct ListNode* next; // 指针到下一个节点
} ListNode;
// 合并函数
ListNode* mergeTwoLists(ListNode* l1, ListNode* l2) {
if (!l1) return l2;
if (!l2) return l1;
// 创建一个新的头节点,并初始化指针
ListNode* head = NULL, *temp = NULL;
if (l1->val <= l2->val) {
head = l1;
temp = l1->next;
l1->next = l2;
} else {
head = l2;
temp = l2->next;
l2->next = l1;
}
while (temp && temp->next) { // 遍历较小链表直到遇到较大链表的结束
if (temp->next->val <= temp->val) {
ListNode* tmp = temp->next;
temp->next = temp->next->next;
tmp->next = temp;
temp = tmp;
} else {
temp = temp->next;
}
}
// 如果较大链表剩余部分未处理,则将其添加到新链表
if (temp) {
temp->next = NULL;
head->next = l2;
} else {
head->next = l1;
}
return head;
}
// 测试示例
void printList(ListNode* node) {
while (node != NULL) {
printf("%d -> ", node->val);
node = node->next;
}
printf("NULL\n");
}
int main() {
// 创建两个示例链表
ListNode* list1 = (ListNode*)malloc(sizeof(ListNode));
list1->val = 1;
list1->next = (ListNode*)malloc(sizeof(ListNode));
list1->next->val = 2;
list1->next->next = (ListNode*)malloc(sizeof(ListNode));
list1->next->next->val = 4;
ListNode* list2 = (ListNode*)malloc(sizeof(ListNode));
list2->val = 1;
list2->next = (ListNode*)malloc(sizeof(ListNode));
list2->next->val = 3;
list2->next->next = (ListNode*)malloc(sizeof(ListNode));
list2->next->next->val = 4;
// 合并并打印结果
ListNode* result = mergeTwoLists(list1, list2);
printList(result);
return 0;
}
```
阅读全文
相关推荐
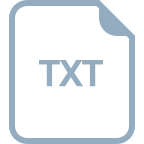
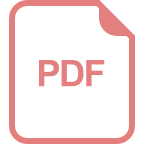
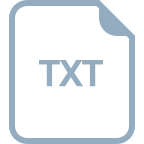
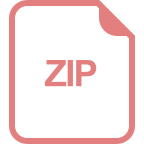
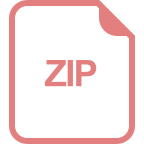
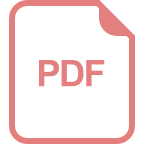
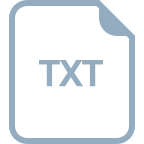
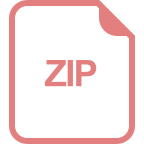
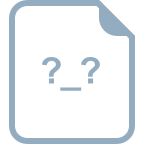
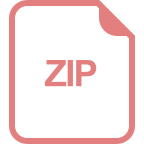
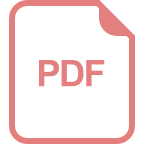
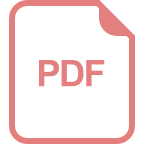